用c语言编写从city.txt文件中读十个城市名字,要求: 自定义一个排序函数sorted( ),在主程序中调用该自定义函数,对所读取的10个城市进行升序排序,并把结果输出在屏幕上。 用户输入一个城市的名字,如果city.txt文件中有这个城市名字,则显示“查到这个城市!”,否则显示“没有找到这个城市!”。
时间: 2024-03-13 16:47:55 浏览: 182
好的,我可以帮你解决这个问题。
首先,我们需要定义一个结构体来存储城市名字:
```c
typedef struct {
char name[50];
} City;
```
然后,我们可以定义一个函数来读取城市名字:
```c
void read_cities(City cities[], int n) {
FILE *fp = fopen("city.txt", "r");
if (fp == NULL) {
printf("Failed to open file!\n");
return;
}
for (int i = 0; i < n; i++) {
fscanf(fp, "%s", cities[i].name);
}
fclose(fp);
}
```
接着,我们可以定义一个排序函数,这里使用冒泡排序:
```c
void sorted(City cities[], int n) {
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (strcmp(cities[j].name, cities[j + 1].name) > 0) {
City tmp = cities[j];
cities[j] = cities[j + 1];
cities[j + 1] = tmp;
}
}
}
}
```
最后,我们可以编写主函数,调用上述函数:
```c
int main() {
City cities[10];
read_cities(cities, 10);
sorted(cities, 10);
printf("Sorted cities:\n");
for (int i = 0; i < 10; i++) {
printf("%s\n", cities[i].name);
}
printf("Please enter a city name: ");
char name[50];
scanf("%s", name);
int found = 0;
for (int i = 0; i < 10; i++) {
if (strcmp(cities[i].name, name) == 0) {
found = 1;
break;
}
}
if (found) {
printf("Found this city!\n");
} else {
printf("Cannot find this city!\n");
}
return 0;
}
```
这样,就可以实现你的需求了。
阅读全文
相关推荐
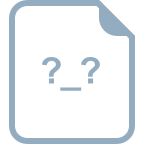










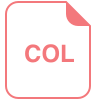




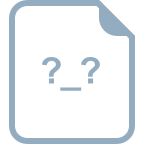