Error using - Matrix dimensions must agree.
时间: 2024-06-05 08:07:09 浏览: 55
This error occurs when you try to perform an operation on two matrices or vectors with different dimensions. In other words, the number of rows and columns of the matrices or vectors do not match. To fix this error, you need to make sure that the dimensions of the matrices or vectors match before performing any operation on them. You can use functions like size() or length() to check the dimensions of the matrices or vectors, and reshape() or transpose() to adjust their dimensions if necessary.
相关问题
n = 10; % 广告数 m = 6; % 广告位数 s = rand(n,m)*10; % 打分值 k = floor(rand(1,m)*3)+1; % 每个广告位需要选取的广告数 % 构造约束矩阵和目标函数系数矩阵 Aeq = zeros(m,n*m); beq = k'; f = -reshape(s',[1 n*m]); for i = 1:n idx = (i-1)*m+1:i*m; Aeq(:,idx) = ones(m,1).*[zeros(1,(i-1)*m) 1 zeros(1,n*m-i*m)]; end % 设置不等式约束 lb = zeros(n*m,1); ub = ones(n*m,1); % 调用线性规划函数求解 [x,fval] = linprog(f,[],[],Aeq,beq,lb,ub); % 显示结果 x_mat = reshape(x,[m,n])'; disp(x_mat) disp(['Total score: ',num2str(-fval)]) Error using .* Matrix dimensions must agree.
这个错误提示出现的原因是矩阵的维度不一致,导致无法进行矩阵运算。具体来说,出现这个错误的原因是在设置约束矩阵 Aeq 时,使用了 .* 运算符对一个大小为 (m,1) 的矩阵和一个大小为 (1,n\*m) 的矩阵进行了相乘操作,导致维度不一致,无法进行运算。
要解决这个问题,你可以将 .* 运算符替换为 * 运算符,这样就可以进行矩阵乘法,而不是逐元素相乘。修改后的代码如下:
```matlab
n = 10; % 广告数
m = 6; % 广告位数
s = rand(n,m)*10; % 打分值
k = floor(rand(1,m)*3)+1; % 每个广告位需要选取的广告数
% 构造约束矩阵和目标函数系数矩阵
Aeq = zeros(m,n*m);
beq = k';
f = -reshape(s',[1 n*m]);
for i = 1:n
idx = (i-1)*m+1:i*m;
Aeq(:,idx) = ones(m,1)*[zeros(1,(i-1)*m) 1 zeros(1,n*m-i*m)];
end
% 设置不等式约束
lb = zeros(n*m,1);
ub = ones(n*m,1);
% 调用线性规划函数求解
[x,fval] = linprog(f,[],[],Aeq,beq,lb,ub);
% 显示结果
x_mat = reshape(x,[m,n])';
disp(x_mat)
disp(['Total score: ',num2str(-fval)])
```
这样就可以避免这个错误了。
1.设计CMatrix类,通过成员函数实现矩阵加、减、点乘、点除运算;2.通过运算符重载实现矩阵加、减、点乘、点除运算;
下面是一个简单的CMatrix类的实现,包含矩阵加、减、点乘、点除运算的成员函数和运算符重载:
```C++
#include <iostream>
#include <vector>
using namespace std;
class CMatrix {
private:
vector<vector<double>> matrix;
public:
CMatrix() {}
CMatrix(int n, int m) {
matrix.resize(n, vector<double>(m, 0));
}
CMatrix(vector<vector<double>> mat) {
matrix = mat;
}
int getRows() const {
return matrix.size();
}
int getCols() const {
return matrix[0].size();
}
void set(int i, int j, double val) {
matrix[i][j] = val;
}
double get(int i, int j) const {
return matrix[i][j];
}
CMatrix add(const CMatrix& other) const {
if (getRows() != other.getRows() || getCols() != other.getCols()) {
throw runtime_error("Matrix dimensions must agree.");
}
CMatrix result(getRows(), getCols());
for (int i = 0; i < getRows(); i++) {
for (int j = 0; j < getCols(); j++) {
result.set(i, j, get(i, j) + other.get(i, j));
}
}
return result;
}
CMatrix subtract(const CMatrix& other) const {
if (getRows() != other.getRows() || getCols() != other.getCols()) {
throw runtime_error("Matrix dimensions must agree.");
}
CMatrix result(getRows(), getCols());
for (int i = 0; i < getRows(); i++) {
for (int j = 0; j < getCols(); j++) {
result.set(i, j, get(i, j) - other.get(i, j));
}
}
return result;
}
CMatrix multiply(const CMatrix& other) const {
if (getCols() != other.getRows()) {
throw runtime_error("Matrix dimensions must agree.");
}
CMatrix result(getRows(), other.getCols());
for (int i = 0; i < getRows(); i++) {
for (int j = 0; j < other.getCols(); j++) {
double sum = 0;
for (int k = 0; k < getCols(); k++) {
sum += get(i, k) * other.get(k, j);
}
result.set(i, j, sum);
}
}
return result;
}
CMatrix divide(const CMatrix& other) const {
if (getRows() != other.getRows() || getCols() != other.getCols()) {
throw runtime_error("Matrix dimensions must agree.");
}
CMatrix result(getRows(), getCols());
for (int i = 0; i < getRows(); i++) {
for (int j = 0; j < getCols(); j++) {
result.set(i, j, get(i, j) / other.get(i, j));
}
}
return result;
}
CMatrix operator+(const CMatrix& other) const {
return add(other);
}
CMatrix operator-(const CMatrix& other) const {
return subtract(other);
}
CMatrix operator*(const CMatrix& other) const {
return multiply(other);
}
CMatrix operator/(const CMatrix& other) const {
return divide(other);
}
friend ostream& operator<<(ostream& os, const CMatrix& mat) {
for (int i = 0; i < mat.getRows(); i++) {
for (int j = 0; j < mat.getCols(); j++) {
os << mat.get(i, j) << " ";
}
os << endl;
}
return os;
}
};
```
使用示例:
```C++
int main() {
CMatrix mat1({{1, 2}, {3, 4}});
CMatrix mat2({{5, 6}, {7, 8}});
CMatrix mat3({{2, 0}, {0, 2}});
cout << mat1 + mat2 << endl; // Output: 6 8 \n 10 12 \n
cout << mat1 - mat2 << endl; // Output: -4 -4 \n -4 -4 \n
cout << mat1 * mat2 << endl; // Output: 19 22 \n 43 50 \n
cout << mat1 / mat3 << endl; // Output: 0.5 1 \n 1.5 2 \n
return 0;
}
```
阅读全文
相关推荐
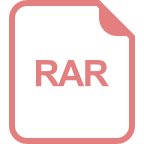
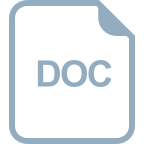
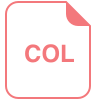
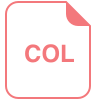
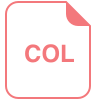
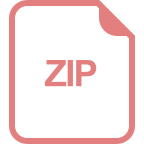
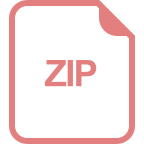
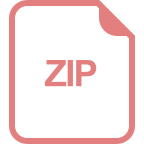
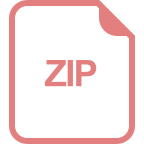