linux打印a.sh文件,忽略空行和注释行
时间: 2024-05-11 12:16:29 浏览: 101
您可以使用以下命令来打印a.sh文件,同时忽略空行和注释行:
```
grep -v '^#' a.sh | grep -v '^$'
```
其中,`grep -v '^#'`用于过滤掉以`#`开头的注释行,`grep -v '^$'`用于过滤掉空行。最终的效果是只打印出a.sh文件中的实际代码行。
相关问题
linux环境下c程序的编写,利用 C 语言编写一个微型命令解释程序,使其能够接收并执行以 下命令: (1) dir 列出当前目录 (2) cop 文件名 1 文件名 2 拷贝文件 (3) era 文件名 删除文件 (4) dis 字符串 显示字符串 (5) end 结束,退出
在Linux环境下,使用C语言编写一个简单的命令解释器,可以按照下面的步骤进行:
首先,创建一个`command_interpreter.c`文件,并定义一个结构体来存储指令、操作和参数:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct {
const char* cmd;
int (*func)(const char*, const char*);
} Command;
Command commands[] = {
{"dir", list_files},
{"cop", copy_files},
{"era", remove_file},
{"dis", display_string},
{"end", exit_program}
};
```
接着,定义对应的函数来处理每个命令:
```c
// 函数列表
int list_files(const char* arg1, const char*) {
// 实现列出当前目录的功能...
}
int copy_files(const char* arg1, const char* arg2) {
// 实现复制文件功能...
}
int remove_file(const char* arg1, const char*) {
// 实现删除文件功能...
}
int display_string(const char* arg1, const char*) {
// 实现显示字符串功能...
}
void exit_program(const char*, const char*) {
printf("结束程序\n");
exit(0);
}
```
然后,在主函数`main()`中读取用户输入,解析命令并调用相应的函数:
```c
int main() {
char input[256];
while (fgets(input, sizeof(input), stdin)) {
if (!input[0] || input[0] == '#') continue; // 忽略空行和注释
char** args = strtok_r(input, " ", &input); // 分割命令和参数
if (!args) break; // 输入结束
for (size_t i = 0; i < sizeof(commands) / sizeof(Command); ++i) {
if (strcmp(args[0], commands[i].cmd) == 0) {
if (commands[i].func(args + 1, args + 2)) {
printf("错误: %s\n", args[0]);
}
break;
}
}
}
return 0;
}
```
最后,记得链接必要的库并编译你的程序:
```sh
gcc -o command_interpreter command_interpreter.c
```
这个例子只是一个基础框架,实际的文件操作需要使用`<unistd.h>`或其他适当的系统调用来完成。
阅读全文
相关推荐
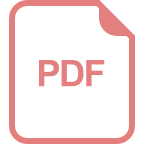
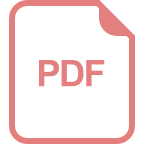
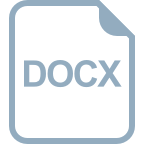
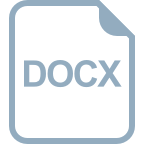
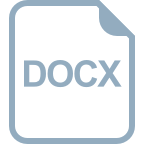
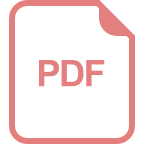
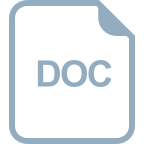
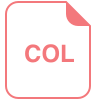
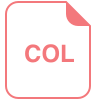
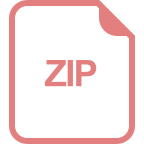
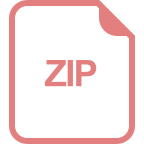
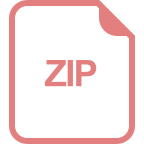
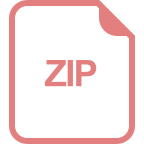
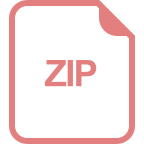