python散点图设置坐标轴范围
时间: 2023-09-12 14:02:37 浏览: 88
使用Matplotlib库可以设置散点图的坐标轴范围。具体操作如下:
1. 导入Matplotlib库
```
import matplotlib.pyplot as plt
```
2. 绘制散点图
```
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
plt.scatter(x, y)
```
3. 设置坐标轴范围
```
plt.xlim(0, 6) # 设置x轴范围
plt.ylim(0, 12) # 设置y轴范围
```
完整代码如下:
```
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
plt.scatter(x, y)
plt.xlim(0, 6)
plt.ylim(0, 12)
plt.show()
```
相关问题
python散点图设置坐标轴标度
在Python中,可以使用matplotlib库来绘制散点图,并设置坐标轴标度。以下是一个示例代码:
```python
import matplotlib.pyplot as plt
# 生成随机数据
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
# 绘制散点图
plt.scatter(x, y)
# 设置x轴标度
plt.xticks([1, 2, 3, 4, 5], ['A', 'B', 'C', 'D', 'E'])
# 设置y轴标度
plt.yticks([2, 4, 6, 8, 10], ['Low', 'Medium', 'High', 'Very High', 'Extreme'])
# 显示图像
plt.show()
```
在上述代码中,我们使用`scatter()`函数绘制散点图,并使用`xticks()`和`yticks()`函数来设置x轴和y轴的标度。每个函数都接受两个参数:第一个参数是标度值的列表,第二个参数是对应的标度标签的列表。例如,`xticks([1, 2, 3, 4, 5], ['A', 'B', 'C', 'D', 'E'])`表示将x轴的标度设置为1、2、3、4、5,并将它们分别替换为A、B、C、D、E。最后,我们调用`show()`函数显示图像。
python画折线图设置坐标轴
在 Python 中,可以使用 Matplotlib 库绘制折线图,并且可以通过设置坐标轴来自定义图表的外观。下面是一些示例代码,演示如何设置坐标轴:
```python
import matplotlib.pyplot as plt
# 生成数据
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
# 绘制折线图
plt.plot(x, y)
# 设置 x 坐标轴
plt.xlim(0, 6) # 设置 x 轴范围
plt.xticks([1, 2, 3, 4, 5]) # 设置 x 轴刻度
# 设置 y 坐标轴
plt.ylim(0, 12) # 设置 y 轴范围
plt.yticks([2, 4, 6, 8, 10]) # 设置 y 轴刻度
# 添加标题和标签
plt.title('Example Line Plot')
plt.xlabel('X Axis')
plt.ylabel('Y Axis')
# 显示图表
plt.show()
```
在这个示例中,我们在绘制折线图之后,使用 `plt.xlim()` 和 `plt.ylim()` 来设置 x 和 y 坐标轴的范围。然后,我们使用 `plt.xticks()` 和 `plt.yticks()` 来设置 x 和 y 轴的刻度。最后,我们使用 `plt.title()`、`plt.xlabel()` 和 `plt.ylabel()` 来添加标题和坐标轴标签。最后,我们调用 `plt.show()` 来显示图表。
相关推荐
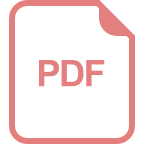
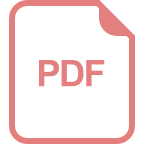
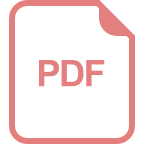












