android 使用mqt
时间: 2023-07-11 20:35:46 浏览: 75
您好,如果您想在 Android 应用程序中使用 MQTT 协议进行消息传输,可以使用 Eclipse Paho Android 客户端库。以下是使用 Paho Android 客户端库实现 MQTT 的基本步骤:
1. 添加 Paho Android 客户端库到您的项目中。
2. 连接到 MQTT 代理服务器。您需要指定代理服务器的地址和端口号。可以使用 `MqttConnectOptions` 类设置连接选项,例如设置连接的用户名和密码、清除会话标志等。
3. 订阅主题。您需要指定要订阅的主题和 QoS 等级。
4. 发布消息。您需要指定要发布的主题、消息内容和 QoS 等级。
5. 处理接收到的消息。您需要实现 `MqttCallback` 接口,并在回调方法中处理接收到的消息。
以下是使用 Paho Android 客户端库连接到 MQTT 代理服务器的示例代码:
```java
String brokerUrl = "tcp://mqtt.eclipse.org:1883";
String clientId = MqttClient.generateClientId();
MqttAndroidClient client = new MqttAndroidClient(context, brokerUrl, clientId);
MqttConnectOptions options = new MqttConnectOptions();
options.setUserName("username");
options.setPassword("password".toCharArray());
options.setCleanSession(true);
client.connect(options);
```
以下是订阅主题和发布消息的示例代码:
```java
String topic = "my/topic";
int qos = 1;
client.subscribe(topic, qos);
String message = "Hello, MQTT!";
client.publish(topic, message.getBytes(), qos, false);
```
您还需要实现 `MqttCallback` 接口并在回调方法中处理接收到的消息。例如:
```java
client.setCallback(new MqttCallback() {
@Override
public void connectionLost(Throwable cause) {
// 处理连接丢失事件
}
@Override
public void messageArrived(String topic, MqttMessage message) throws Exception {
// 处理接收到的消息
String payload = new String(message.getPayload());
Log.d(TAG, "Received message: " + payload);
}
@Override
public void deliveryComplete(IMqttDeliveryToken token) {
// 处理消息发送完成事件
}
});
```
希望这些信息能对您有所帮助。
相关推荐





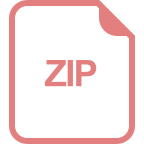
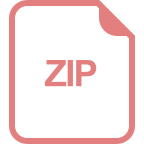
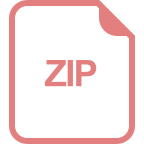
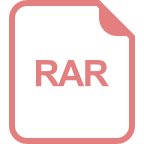
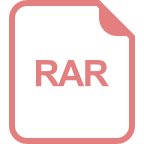
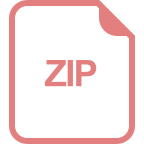