1.Write a Java program called ArithmeticT1 that takes three command-line arguments: two integers and in between an arithmetic operator (+, ‐, *, /). The program shall perform the corresponding operation on the two integers and display the result. Note that you need to deal with the nonnumeric input as operands (one of the two integers) using exception handler (try-catch statements) - the program terminates if any operand is nonnumeric, and it should display a message with the wrong operand input before exiting. Besides, the program should also handle the wrong input for operator.
时间: 2024-03-11 22:43:31 浏览: 120
下面是Java程序,用于执行两个整数之间的算术运算,并处理非数字操作数和错误输入操作符:
```java
public class ArithmeticT1 {
public static void main(String[] args) {
try {
int num1 = Integer.parseInt(args[0]);
int num2 = Integer.parseInt(args[1]);
char operator = args[2].charAt(0);
int result = 0;
switch (operator) {
case '+':
result = num1 + num2;
break;
case '-':
result = num1 - num2;
break;
case '*':
result = num1 * num2;
break;
case '/':
result = num1 / num2;
break;
default:
throw new IllegalArgumentException("Invalid operator: " + operator);
}
System.out.println(num1 + " " + operator + " " + num2 + " = " + result);
} catch (NumberFormatException e) {
System.out.println("Error: Non-numeric operand provided.");
} catch (IllegalArgumentException e) {
System.out.println("Error: " + e.getMessage());
}
}
}
```
该程序使用了try-catch语句来处理非数字操作数和错误输入操作符。如果非数字操作数被提供,程序将抛出NumberFormatException异常并显示错误消息。如果输入了错误的操作符,程序将抛出IllegalArgumentException异常并显示错误消息。否则,程序将执行相应的算术运算,并显示结果。
阅读全文
相关推荐
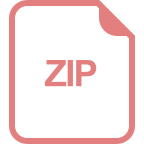
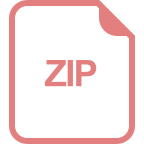
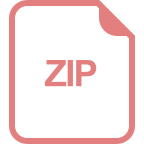
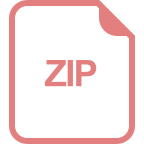
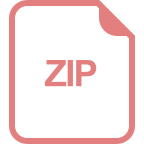
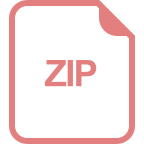
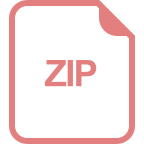
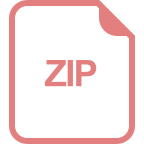
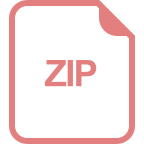
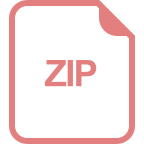
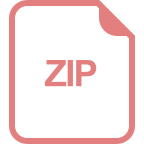
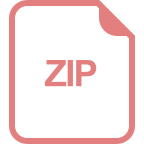
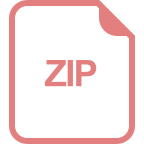
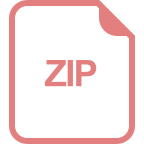
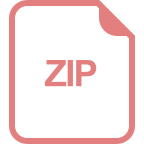