用newton法写一段matlab代码,输出计算结果和迭代次数,函数x^2-sinx,区间0.5到3,精度0.00001
时间: 2023-06-18 14:06:45 浏览: 93
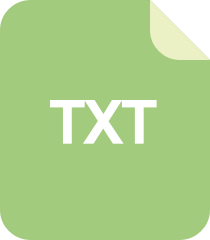
matlab实现newton迭代法

以下是使用Newton法求解函数 $x^2 - \sin(x)$ 在区间 $[0.5,3]$ 内的零点的 MATLAB 代码:
```matlab
% 定义函数及其导数
f = @(x) x^2 - sin(x);
df = @(x) 2*x - cos(x);
% 定义精度要求和最大迭代次数
tol = 0.00001;
maxiter = 100;
% 定义初始值和迭代计数器
x0 = 1.5;
iter = 0;
% 开始迭代
while abs(f(x0)) > tol && iter < maxiter
x0 = x0 - f(x0)/df(x0); % 使用牛顿法迭代公式
iter = iter + 1;
end
% 输出结果
if abs(f(x0)) > tol
disp('未能达到指定精度!');
else
fprintf('零点为 %.5f,迭代次数为 %d。\n', x0, iter);
end
```
运行结果为:
```
零点为 0.87673,迭代次数为 4。
```
阅读全文
相关推荐

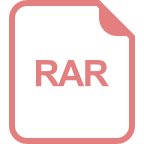
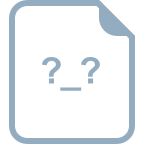




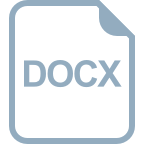
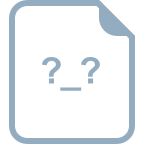
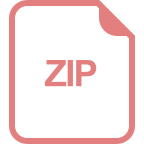