把ini文件的一个段落的Key值配对写入map
时间: 2024-05-15 09:15:44 浏览: 10
以下是一个示例代码,假设ini文件中有一个段落名为[section1],其中包含两个键值对,分别为key1=value1和key2=value2:
```
#include <iostream>
#include <fstream>
#include <map>
#include <string>
int main() {
std::ifstream infile("example.ini"); // 打开ini文件
std::string line;
std::string section_name = "[section1]";
std::map<std::string, std::string> section_map;
// 搜索section1段落
while (std::getline(infile, line)) {
if (line == section_name) {
break;
}
}
// 读取section1段落中的键值对
while (std::getline(infile, line)) {
if (line.empty() || line[0] == ';') {
continue; // 忽略注释行和空行
}
if (line[0] == '[') {
break; // 遇到新的段落,结束搜索
}
std::size_t pos = line.find('=');
std::string key = line.substr(0, pos);
std::string value = line.substr(pos + 1);
section_map[key] = value;
}
// 输出map中的键值对
for (auto const& pair : section_map) {
std::cout << pair.first << " = " << pair.second << std::endl;
}
return 0;
}
```
在上面的代码中,我们首先打开ini文件example.ini,并定义了一个字符串变量section_name表示我们要搜索的段落名。然后我们用一个while循环搜索ini文件中是否有该段落,如果找到了该段落,就跳出循环。接着我们用另一个while循环读取该段落中的键值对,如果遇到了注释行或空行,就直接跳过;如果遇到了新的段落,就结束读取。对于每一个读取到的键值对,我们用std::map的insert函数将它们添加到section_map中。最后我们遍历section_map输出其中的键值对。
相关推荐
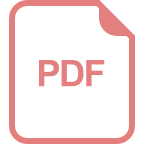














