怎么把JLabel中的图像写入文件,再次打开文件能读取出来
时间: 2024-02-18 08:03:28 浏览: 19
要将JLabel中的图像写入文件并再次打开文件能读取出来,可以使用Java的序列化机制。Java的序列化可以将对象转换为字节序列,然后将字节序列保存到文件中,再次读取时可以将字节序列恢复为原来的对象。因此,我们可以将JLabel中的图像对象序列化为字节序列,然后将字节序列写入文件,再次读取时将字节序列反序列化为图像对象,然后将图像对象设置到JLabel中。
下面是一个示例代码:
```java
// 将JLabel中的图像对象序列化为字节序列并写入到文件中
try {
ImageIcon icon = (ImageIcon) label.getIcon();
BufferedImage image = new BufferedImage(icon.getIconWidth(), icon.getIconHeight(), BufferedImage.TYPE_INT_RGB);
Graphics g = image.createGraphics();
icon.paintIcon(null, g, 0, 0);
g.dispose();
ByteArrayOutputStream baos = new ByteArrayOutputStream();
ObjectOutputStream oos = new ObjectOutputStream(baos);
oos.writeObject(image);
byte[] bytes = baos.toByteArray();
FileOutputStream fos = new FileOutputStream("label_image.ser");
fos.write(bytes);
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
// 从文件中读取字节序列并反序列化为图像对象,然后设置到JLabel中
try {
FileInputStream fis = new FileInputStream("label_image.ser");
byte[] bytes = fis.readAllBytes();
fis.close();
ByteArrayInputStream bais = new ByteArrayInputStream(bytes);
ObjectInputStream ois = new ObjectInputStream(bais);
BufferedImage image = (BufferedImage) ois.readObject();
ois.close();
JLabel label = new JLabel(new ImageIcon(image));
} catch (IOException | ClassNotFoundException e) {
e.printStackTrace();
}
```
这样就能将JLabel中的图像对象写入文件并恢复出来了。需要注意的是,这里序列化和反序列化的对象是BufferedImage而不是JLabel,因为BufferedImage才是图像对象的实际类型。
相关推荐
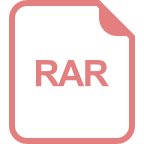
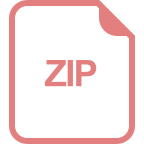
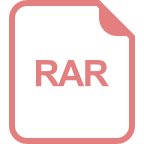














