springboot将redis的哈希数据同步到MySQL库写出service层,controller层,dao层
时间: 2023-05-27 15:01:48 浏览: 133
注:以下代码仅为示例,实际应用中需要根据具体业务进行相应的调整和完善。
**1. Service层代码:**
```
public interface RedisHashSyncService {
/**
* 从Redis中获取指定哈希表的所有键值对,并将其同步到MySQL中
*
* @param hashKey Redis哈希表的key值
* @return 同步的记录数
*/
int syncRedisHashToMysql(String hashKey);
}
```
```
@Service
public class RedisHashSyncServiceImpl implements RedisHashSyncService {
private final RedisTemplate<String, Object> redisTemplate;
private final RedisHashSyncMapper redisHashSyncMapper;
@Autowired
public RedisHashSyncServiceImpl(RedisTemplate<String, Object> redisTemplate,
RedisHashSyncMapper redisHashSyncMapper) {
this.redisTemplate = redisTemplate;
this.redisHashSyncMapper = redisHashSyncMapper;
}
@Override
public int syncRedisHashToMysql(String hashKey) {
// 从Redis中获取指定哈希表的所有键值对
Map<Object, Object> redisMap = redisTemplate.opsForHash().entries(hashKey);
// 遍历Map中的所有键值对,将其同步到MySQL中
int count = 0;
for (Map.Entry<Object, Object> entry : redisMap.entrySet()) {
RedisHashSyncDO redisHashSyncDO = new RedisHashSyncDO();
redisHashSyncDO.setHashKey(hashKey);
redisHashSyncDO.setHashField(entry.getKey().toString());
redisHashSyncDO.setHashValue(entry.getValue().toString());
redisHashSyncMapper.insert(redisHashSyncDO);
count++;
}
return count;
}
}
```
**2. Controller层代码:**
```
@RestController
@RequestMapping("/redis")
public class RedisController {
private final RedisHashSyncService redisHashSyncService;
@Autowired
public RedisController(RedisHashSyncService redisHashSyncService) {
this.redisHashSyncService = redisHashSyncService;
}
@PostMapping("/sync/hash")
public ResponseEntity<String> syncRedisHashToMysql(@RequestParam("hashKey") String hashKey) {
int count = redisHashSyncService.syncRedisHashToMysql(hashKey);
return ResponseEntity.ok().body("Sync " + count + " records from Redis hash " + hashKey + " to MySQL.");
}
}
```
**3. Dao层代码:**
```
@Mapper
public interface RedisHashSyncMapper {
/**
* 向MySQL中插入一条Redis哈希表数据同步记录
*
* @param redisHashSyncDO Redis哈希表数据同步记录对象
* @return 操作影响的行数
*/
int insert(RedisHashSyncDO redisHashSyncDO);
}
```
```
@Data
public class RedisHashSyncDO {
/**
* 主键id
*/
private Integer id;
/**
* Redis哈希表的key值
*/
private String hashKey;
/**
* Redis哈希表的field值
*/
private String hashField;
/**
* Redis哈希表的value值
*/
private String hashValue;
/**
* 创建时间
*/
private Date createTime;
/**
* 更新时间
*/
private Date updateTime;
}
```
阅读全文
相关推荐




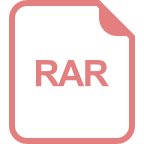










