C#web应用程序查询数据库数据显示为折线图后端怎么写
时间: 2024-02-23 07:02:06 浏览: 62
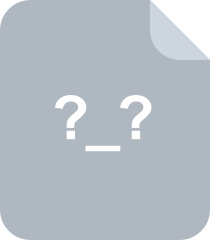
C#实现折线图

在 C# 的 Web 应用程序中,可以使用 ASP.NET MVC 框架和 Chart.js 库来实现查询数据库数据并显示为折线图。下面是一个简单的示例:
1. 在 Model 中定义数据模型,例如:
```
public class SalesData
{
public DateTime Date { get; set; }
public int Revenue { get; set; }
}
```
2. 在 Controller 中编写查询数据库数据的代码,例如:
```
public ActionResult SalesChart()
{
List<SalesData> salesDataList = new List<SalesData>();
string connectionString = "Data Source=your_server_name;Initial Catalog=your_database_name;User ID=your_user_id;Password=your_password;";
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
SqlCommand command = new SqlCommand("SELECT Date, Revenue FROM Sales", connection);
SqlDataReader reader = command.ExecuteReader();
while (reader.Read())
{
SalesData salesData = new SalesData();
salesData.Date = reader.GetDateTime(0);
salesData.Revenue = reader.GetInt32(1);
salesDataList.Add(salesData);
}
reader.Close();
}
return View(salesDataList);
}
```
3. 在 View 中使用 Chart.js 库来绘制折线图,例如:
```
@model List<SalesData>
<script src="https://cdn.jsdelivr.net/npm/chart.js"></script>
<canvas id="salesChart"></canvas>
<script>
var salesData = @Html.Raw(Json.Encode(Model.Select(x => new { Date = x.Date.ToString("yyyy-MM-dd"), Revenue = x.Revenue })));
var salesChart = new Chart(document.getElementById("salesChart"), {
type: 'line',
data: {
labels: salesData.map(x => x.Date),
datasets: [{
label: 'Sales',
data: salesData.map(x => x.Revenue),
borderColor: 'blue',
fill: false
}]
},
options: {
responsive: true,
legend: {
display: false
},
scales: {
xAxes: [{
type: 'time',
time: {
unit: 'day'
}
}],
yAxes: [{
ticks: {
beginAtZero: true
}
}]
}
}
});
</script>
```
这样就可以在 Web 应用程序中查询数据库数据并显示为折线图了。当然,具体的实现方式还需要根据你的具体需求来做相应的调整。
阅读全文
相关推荐
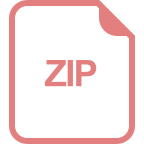
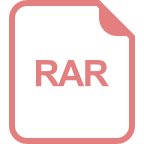
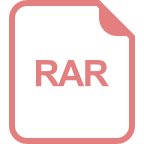
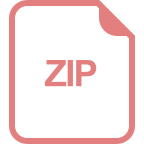
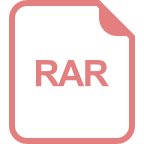
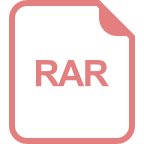
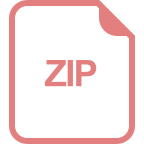
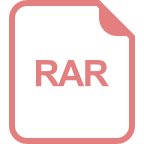
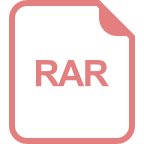
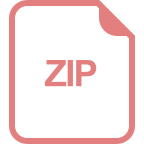
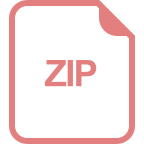
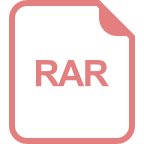
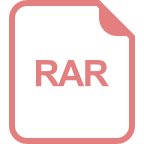
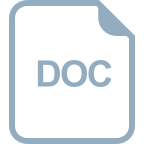
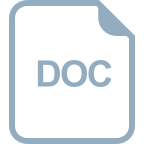
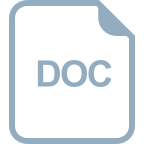
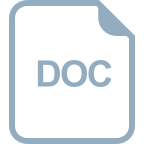
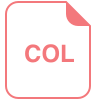