用switch写出一个简易循环
时间: 2024-05-02 10:20:57 浏览: 11
以下是用switch语句编写的简易循环示例:
```
int i = 0;
while (i < 5) {
switch (i) {
case 0:
printf("i is zero\n");
break;
case 1:
printf("i is one\n");
break;
case 2:
printf("i is two\n");
break;
case 3:
printf("i is three\n");
break;
case 4:
printf("i is four\n");
break;
}
i++;
}
```
输出:
```
i is zero
i is one
i is two
i is three
i is four
```
在这个示例中,我们使用了一个while循环来遍历i变量的值,从0到4。在每个迭代中,我们使用switch语句来检查i的值,并输出相应的消息。最后,我们增加i的值以继续迭代,直到i达到5。
相关问题
用Switch语句写一个死循环
可以这样写:
```
while (true) {
switch (1) {
case 1:
// do something
break;
default:
// do something else
break;
}
}
```
这里使用了一个永远为真的 while 循环,以及一个 switch 语句来执行不同的操作。由于 case 1 永远为真,因此程序会一直执行其中的代码直到被强制结束。
java用switch写一个简易四则运算
好的,以下是一个简易四则运算的Java代码,使用switch语句:
```java
import java.util.Scanner;
public class SimpleCalculator {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入第一个数:");
double num1 = scanner.nextDouble();
System.out.print("请输入第二个数:");
double num2 = scanner.nextDouble();
System.out.print("请输入运算符(+、-、*、/):");
char operator = scanner.next().charAt(0);
double result = 0.0;
switch(operator) {
case '+':
result = num1 + num2;
break;
case '-':
result = num1 - num2;
break;
case '*':
result = num1 * num2;
break;
case '/':
result = num1 / num2;
break;
default:
System.out.println("错误的运算符");
return;
}
System.out.println("运算结果:" + result);
}
}
```
运行程序后,按照提示输入两个数和运算符,即可得到运算结果。注意要进行异常处理,例如输入的运算符不是四则运算符时,要给出错误提示。
相关推荐
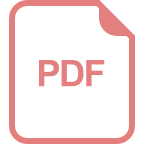












