写一个android app界面布局 高级组件 后台服务和广播 多媒体
时间: 2024-02-05 10:04:42 浏览: 83
好的,下面是一个简单的 Android 应用程序,包含界面布局、高级组件、后台服务、广播和多媒体:
1. 界面布局:
使用相对布局和线性布局来创建一个简单的界面布局,其中包括一个文本输入框、一个按钮和一个文本视图。用户可以在文本输入框中输入文本,点击按钮后,文本视图将显示用户输入的文本。XML 代码如下:
```
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<EditText
android:id="@+id/editText"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Enter text here"
android:padding="16dp" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@id/editText"
android:layout_centerHorizontal="true"
android:layout_marginTop="16dp"
android:text="Submit" />
<TextView
android:id="@+id/textView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/button"
android:padding="16dp" />
</RelativeLayout>
```
2. 高级组件:
使用 RecyclerView 组件来展示一个列表,其中包括多个文本视图和图像视图。使用 LinearLayoutManager 来实现线性布局,使用 RecyclerView.Adapter 来绑定数据。Java 代码如下:
```
RecyclerView recyclerView = findViewById(R.id.recyclerView);
recyclerView.setLayoutManager(new LinearLayoutManager(this));
recyclerView.setAdapter(new MyAdapter(data));
```
3. 后台服务:
使用 IntentService 组件来实现后台服务,其中包括一个长时间运行的任务,例如下载文件或处理数据。Java 代码如下:
```
public class MyService extends IntentService {
public MyService() {
super("MyService");
}
@Override
protected void onHandleIntent(@Nullable Intent intent) {
// Perform long-running operation here
}
}
```
4. 广播:
使用 BroadcastReceiver 组件来接收系统广播,例如网络状态变化、电池状态变化等。Java 代码如下:
```
public class MyReceiver extends BroadcastReceiver {
@Override
public void onReceive(Context context, Intent intent) {
// Handle broadcast event here
}
}
```
5. 多媒体:
使用 MediaPlayer 组件来实现音频播放,例如播放背景音乐或语音提示。Java 代码如下:
```
MediaPlayer mediaPlayer = MediaPlayer.create(this, R.raw.sound);
mediaPlayer.start();
```
完成上述步骤后,可以将应用程序打包成 APK 文件,然后安装到 Android 设备上进行测试和发布。当然,这只是一个简单的框架和实现流程,具体的应用程序还需要根据实际需求进行定制和优化。
阅读全文
相关推荐












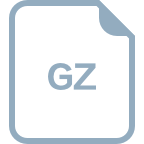



