将C语言源程序前加上行号
时间: 2023-06-12 10:02:30 浏览: 188
以下是一个简单的实现,可以将输入的C语言源程序前面加上行号:
```c
#include <stdio.h>
int main() {
char line[100];
int lineNumber = 1;
// 从标准输入读取每一行,并添加行号
while (fgets(line, sizeof(line), stdin)) {
printf("%d: %s", lineNumber, line);
lineNumber++;
}
return 0;
}
```
在这个程序中,我们使用了`fgets()`函数来从标准输入读取每一行输入,并使用`printf()`函数将行号和原始输入一起输出。请注意,这个程序没有做任何错误处理,例如输入行超过100个字符的情况。如果需要更严格的错误处理,需要进行相应的修改。
相关问题
写一个C语言程序将合法C源程序每行前加上行号并删除其所有注释
当然可以,这是一个常见的需求。下面是一个简单的实现:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LINE_LEN 1024
int main(int argc, char **argv) {
if (argc != 2) {
printf("Usage: addline input_file\n");
return 1;
}
FILE *input_file = fopen(argv[1], "r");
if (input_file == NULL) {
printf("Cannot open file %s\n", argv[1]);
return 2;
}
char *line_buf = malloc(sizeof(char) * MAX_LINE_LEN);
if (line_buf == NULL) {
printf("Cannot allocate memory for line buffer\n");
return 3;
}
char *comment_start;
int line_num = 1;
while (fgets(line_buf, MAX_LINE_LEN, input_file) != NULL) {
// Remove trailing newline character
line_buf[strcspn(line_buf, "\r\n")] = '\0';
// Find start of comment and remove it
comment_start = strstr(line_buf, "//");
if (comment_start != NULL) {
*comment_start = '\0';
}
// Print line with line number
printf("%d: %s\n", line_num, line_buf);
line_num++;
}
free(line_buf);
fclose(input_file);
return 0;
}
```
这个程序读入一个 C 语言源文件,输出一个带行号的版本,并且删除每行的注释。几点说明:
- 程序使用了 fgets 函数逐行读入源程序。MAX_LINE_LEN 宏定义了一行最多能包含的字符数。
- 当遇到注释时,程序通过 strstr 函数找到注释的位置,然后将它后面的部分截掉。这里只考虑了单行注释,如果源程序中还有多行注释、字符常量或字符串,还需要对其进行识别和处理。
- 最后程序使用 printf 输出带行号的源程序。
用C语言编写程序将合法C源程序文件,每行前加上行号并删除其所有注释,存储为新文件。
以下是一个简单的实现,该程序假设输入的C源文件名为input.c,输出的文件名为output.c:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main() {
FILE *fp_in, *fp_out;
fp_in = fopen("input.c", "r");
if (fp_in == NULL) {
printf("Failed to open input file\n");
exit(1);
}
fp_out = fopen("output.c", "w");
if (fp_out == NULL) {
printf("Failed to open output file\n");
exit(1);
}
char line[1024];
int line_num = 1;
while (fgets(line, 1024, fp_in)) {
// Remove comments
char *pos = strstr(line, "//");
if (pos != NULL) {
*pos = '\0';
}
pos = strstr(line, "/*");
if (pos != NULL) {
*pos = '\0';
char *end = strstr(pos + 2, "*/");
if (end != NULL) {
strcpy(pos, end + 2);
}
}
// Add line number
fprintf(fp_out, "%d: %s", line_num, line);
line_num++;
}
fclose(fp_in);
fclose(fp_out);
printf("Done\n");
return 0;
}
```
该程序首先打开输入文件input.c和输出文件output.c,然后逐行读取输入文件中的内容。对于每一行,它会先尝试删除以“//”开头的注释,然后尝试删除以“/*”开头、以“*/”结尾的注释,最后在每一行前添加行号并写入输出文件中。
请注意,这只是一个简单的实现,可能无法处理所有情况。例如,它无法处理多行注释在一行的情况。在实际使用中,您可能需要更复杂的算法来处理这些情况。
阅读全文
相关推荐
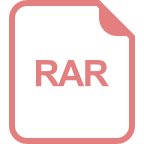
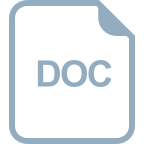
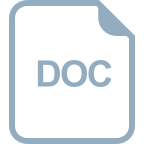



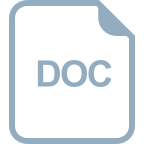
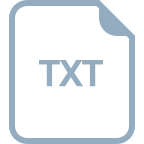
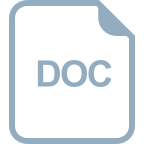
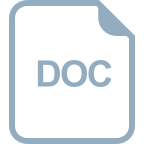
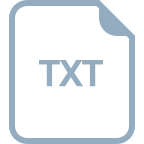
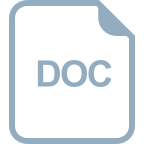



