C# 怎样判断 datagridview 中的checkbox列是否被选中
时间: 2023-08-24 17:33:42 浏览: 197
你可以使用以下代码来判断 DataGridView 中的 CheckBox 是否被选中:
```csharp
bool isChecked = false;
foreach (DataGridViewRow row in dataGridView.Rows)
{
DataGridViewCheckBoxCell checkBoxCell = row.Cells["checkBoxColumnName"] as DataGridViewCheckBoxCell;
if (checkBoxCell.Value != null && (bool)checkBoxCell.Value)
{
isChecked = true;
break;
}
}
```
其中,`"checkBoxColumnName"` 指的是 DataGridView 中 CheckBox 列的列名。这段代码会遍历 DataGridView 中的每一行,获取 CheckBox 单元格的值,如果有任意一个 CheckBox 被选中,就将 `isChecked` 设为 `true`。
相关问题
c# dataGridView的checkbox列实现全选
要实现 DataGridView 中的 checkbox 列实现全选功能,可以按照以下步骤进行:
1. 在 DataGridView 中添加一个 checkbox 列,并设置其 DataPropertyName 属性为数据集中对应的列名。
2. 在 DataGridView 的 DataBindingComplete 事件中,添加以下代码:
```
private void dataGridView1_DataBindingComplete(object sender, DataGridViewBindingCompleteEventArgs e)
{
// 添加全选 checkbox
DataGridViewCheckBoxColumn checkBoxColumn = new DataGridViewCheckBoxColumn();
checkBoxColumn.HeaderText = "全选";
checkBoxColumn.Name = "checkAll";
dataGridView1.Columns.Insert(0, checkBoxColumn);
// 设置全选 checkbox 状态
foreach (DataGridViewRow row in dataGridView1.Rows)
{
row.Cells["checkAll"].Value = false;
}
}
```
3. 在全选 checkbox 的 CellContentClick 事件中,添加以下代码:
```
private void dataGridView1_CellContentClick(object sender, DataGridViewCellEventArgs e)
{
if (e.RowIndex >= 0 && e.ColumnIndex == 0)
{
bool checkAll = (bool)dataGridView1.Rows[e.RowIndex].Cells["checkAll"].Value;
foreach (DataGridViewRow row in dataGridView1.Rows)
{
row.Cells["checkboxColumnName"].Value = checkAll;
}
}
}
```
其中,"checkboxColumnName" 是 checkbox 列对应的列名。
这样,当点击全选 checkbox 时,所有的 checkbox 列都会被选中或取消选中。
C#datagridview中判断checkbox
在C#的DataGridView中判断checkbox的状态,可以通过以下几种方法实现。
方法一:遍历DataGridView的每一行,判断每一行的checkbox是否被选中。可以使用循环来遍历每一行,并通过Cells属性来获取每一行的checkbox单元格的值。例如,可以使用以下代码来判断checkbox的状态:
```csharp
for (int i = 0; i < dataGridView_tabPage1.Rows.Count; i++)
{
bool isChecked = (bool)dataGridView_tabPage1.Rows\[i\].Cells\[0\].Value;
if (isChecked)
{
// checkbox被选中的处理逻辑
}
else
{
// checkbox未被选中的处理逻辑
}
}
```
方法二:使用事件处理程序来判断checkbox的状态。可以为DataGridView的CellValueChanged事件添加事件处理程序,在事件处理程序中判断checkbox的状态。例如,可以使用以下代码来判断checkbox的状态:
```csharp
private void dataGridView_tabPage1_CellValueChanged(object sender, DataGridViewCellEventArgs e)
{
if (e.ColumnIndex == 0 && e.RowIndex >= 0)
{
bool isChecked = (bool)dataGridView_tabPage1.Rows\[e.RowIndex\].Cells\[0\].Value;
if (isChecked)
{
// checkbox被选中的处理逻辑
}
else
{
// checkbox未被选中的处理逻辑
}
}
}
```
以上是两种常用的方法来判断DataGridView中checkbox的状态。根据你的具体需求,选择适合的方法来判断checkbox的状态即可。\[1\]\[2\]\[3\]
#### 引用[.reference_title]
- *1* *2* *3* [Datagridview 添加checkbox列,并判断Datagridview 中的checkbox列是否被选中](https://blog.csdn.net/fangyuan621/article/details/118156656)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insertT0,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
阅读全文
相关推荐
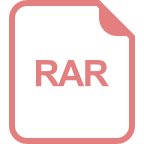
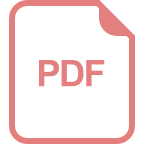
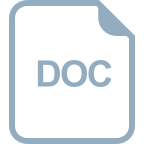
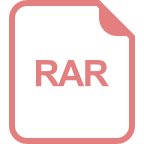
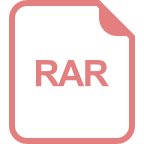
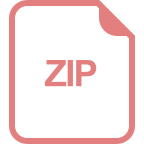
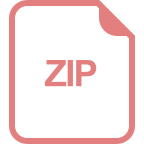
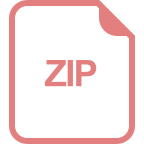
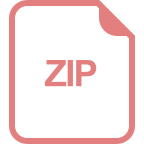
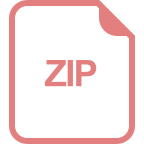
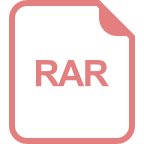
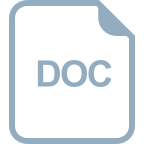


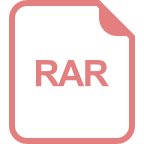
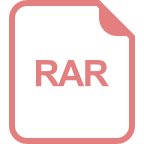