c语言编写程序根据二叉树中序遍历的非递归算法,在二叉树t查找值为x的元素,若找到且其左子树为空,则将值为y的元素插入成为其左孩子,否则若其右孩子为空,则将y插入成为其右孩子。插入失败,返回值为0,否则返回值为1。
时间: 2024-03-14 19:45:32 浏览: 23
好的,以下是用C语言实现上述算法的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
// 定义二叉树节点结构体
typedef struct TreeNode {
int val;
struct TreeNode* left;
struct TreeNode* right;
} TreeNode;
// 定义栈结构体
typedef struct Stack {
int top;
int capacity;
TreeNode** array;
} Stack;
// 初始化栈
Stack* createStack(int capacity) {
Stack* stack = (Stack*) malloc(sizeof(Stack));
stack->top = -1;
stack->capacity = capacity;
stack->array = (TreeNode**) malloc(stack->capacity * sizeof(TreeNode*));
return stack;
}
// 判断栈是否为空
bool isEmpty(Stack* stack) {
return stack->top == -1;
}
// 判断栈是否已满
bool isFull(Stack* stack) {
return stack->top == stack->capacity - 1;
}
// 入栈
void push(Stack* stack, TreeNode* node) {
if (isFull(stack)) {
return;
}
stack->array[++stack->top] = node;
}
// 出栈
TreeNode* pop(Stack* stack) {
if (isEmpty(stack)) {
return NULL;
}
return stack->array[stack->top--];
}
// 非递归中序遍历二叉树
bool inorderTraversal(TreeNode* root, int x, int y) {
Stack* stack = createStack(100);
TreeNode* current = root;
bool flag = false; // 标记是否找到目标节点
while (current != NULL || !isEmpty(stack)) {
while (current != NULL) {
push(stack, current);
current = current->left;
}
current = pop(stack);
if (current->val == x) { // 找到目标节点
if (current->left == NULL) { // 左子树为空,插入y作为左孩子
TreeNode* node = (TreeNode*) malloc(sizeof(TreeNode));
node->val = y;
node->left = NULL;
node->right = NULL;
current->left = node;
flag = true;
} else if (current->right == NULL) { // 右子树为空,插入y作为右孩子
TreeNode* node = (TreeNode*) malloc(sizeof(TreeNode));
node->val = y;
node->left = NULL;
node->right = NULL;
current->right = node;
flag = true;
}
break;
}
current = current->right;
}
return flag;
}
int main() {
// 构造二叉树
TreeNode* t = (TreeNode*) malloc(sizeof(TreeNode));
t->val = 5;
t->left = (TreeNode*) malloc(sizeof(TreeNode));
t->left->val = 3;
t->left->left = (TreeNode*) malloc(sizeof(TreeNode));
t->left->left->val = 2;
t->left->left->left = NULL;
t->left->left->right = NULL;
t->left->right = (TreeNode*) malloc(sizeof(TreeNode));
t->left->right->val = 4;
t->left->right->left = NULL;
t->left->right->right = NULL;
t->right = (TreeNode*) malloc(sizeof(TreeNode));
t->right->val = 7;
t->right->left = (TreeNode*) malloc(sizeof(TreeNode));
t->right->left->val = 6;
t->right->left->left = NULL;
t->right->left->right = NULL;
t->right->right = (TreeNode*) malloc(sizeof(TreeNode));
t->right->right->val = 8;
t->right->right->left = NULL;
t->right->right->right = NULL;
// 测试插入操作
int x = 2, y = 1;
bool result = inorderTraversal(t, x, y);
if (result) {
printf("插入成功!\n");
} else {
printf("插入失败!\n");
}
return 0;
}
```
以上代码中,我们首先定义了二叉树节点结构体和栈结构体,并实现了栈的基本操作。然后,我们使用非递归方式实现了中序遍历二叉树,并在遍历过程中进行节点查找和插入操作。最后,我们通过构造的二叉树和目标值x、插入值y进行了测试。
相关推荐
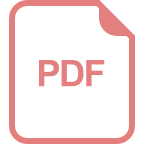
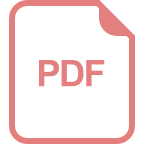
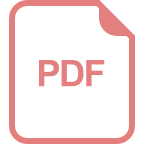
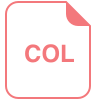













