使用openssl编写限制软件试用期的C++函数 ,对生成的配置文件进行加密以及锁定,无法对其删除,,提示剩余使用时间
时间: 2024-03-27 15:34:51 浏览: 18
您可以使用 OpenSSL 库中的加密算法对配置文件进行加密和解密。以下是一个简单的示例代码:
```C++
#include <openssl/evp.h>
#include <openssl/rand.h>
#include <fstream>
// 加密函数
void encrypt(const std::string& input, const std::string& output, const std::string& password) {
EVP_CIPHER_CTX *ctx = EVP_CIPHER_CTX_new();
// 初始化加密上下文
EVP_EncryptInit_ex(ctx, EVP_aes_256_cbc(), NULL, (const unsigned char*)password.c_str(), (const unsigned char*)"0123456789012345");
std::ifstream in(input.c_str(), std::ios::binary);
std::ofstream out(output.c_str(), std::ios::binary);
// 循环加密数据块
char inbuf[4096];
char outbuf[4096 + EVP_MAX_BLOCK_LENGTH];
int inlen, outlen;
while (in.good()) {
in.read(inbuf, 4096);
inlen = in.gcount();
if (inlen <= 0) break;
// 加密数据块
EVP_EncryptUpdate(ctx, (unsigned char*)outbuf, &outlen, (unsigned char*)inbuf, inlen);
out.write(outbuf, outlen);
}
// 加密结束
EVP_EncryptFinal_ex(ctx, (unsigned char*)outbuf, &outlen);
out.write(outbuf, outlen);
EVP_CIPHER_CTX_free(ctx);
in.close();
out.close();
}
// 解密函数
void decrypt(const std::string& input, const std::string& output, const std::string& password) {
EVP_CIPHER_CTX *ctx = EVP_CIPHER_CTX_new();
// 初始化解密上下文
EVP_DecryptInit_ex(ctx, EVP_aes_256_cbc(), NULL, (const unsigned char*)password.c_str(), (const unsigned char*)"0123456789012345");
std::ifstream in(input.c_str(), std::ios::binary);
std::ofstream out(output.c_str(), std::ios::binary);
// 循环解密数据块
char inbuf[4096];
char outbuf[4096 + EVP_MAX_BLOCK_LENGTH];
int inlen, outlen;
while (in.good()) {
in.read(inbuf, 4096);
inlen = in.gcount();
if (inlen <= 0) break;
// 解密数据块
EVP_DecryptUpdate(ctx, (unsigned char*)outbuf, &outlen, (unsigned char*)inbuf, inlen);
out.write(outbuf, outlen);
}
// 解密结束
EVP_DecryptFinal_ex(ctx, (unsigned char*)outbuf, &outlen);
out.write(outbuf, outlen);
EVP_CIPHER_CTX_free(ctx);
in.close();
out.close();
}
```
您可以在程序启动时读取加密的配置文件,如果未过期,则将其解密,并在程序中使用。如果已经过期,则提示用户剩余使用时间。
您还可以将配置文件的路径存储在程序中,使用系统 APIs (如 Windows 的 CreateFile() 和 SetFileAttributes())将其设置为只读和系统文件,这样用户就无法删除或修改它。
相关推荐
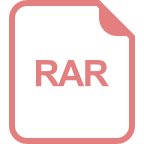
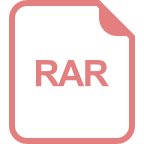












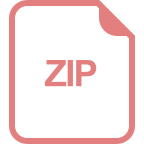
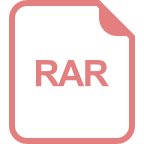
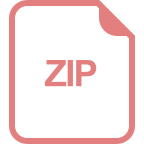