python代码大全游戏
时间: 2023-07-22 21:35:56 浏览: 188
以下是一些基于 Python 的游戏代码示例:
1. 猜数字游戏:
```python
import random
num = random.randint(1, 100)
guess = int(input("请输入一个 1 到 100 之间的整数:"))
while guess != num:
if guess < num:
print("太小了!再试一次。")
else:
print("太大了!再试一次。")
guess = int(input())
print("恭喜你,猜对了!")
```
2. 井字棋游戏:
```python
board = [" " for i in range(9)]
def print_board():
row1 = "|{}|{}|{}|".format(board[0], board[1], board[2])
row2 = "|{}|{}|{}|".format(board[3], board[4], board[5])
row3 = "|{}|{}|{}|".format(board[6], board[7], board[8])
print()
print(row1)
print(row2)
print(row3)
print()
def player_move(icon):
if icon == "X":
number = 1
elif icon == "O":
number = 2
print("你的回合,玩家 {}".format(number))
choice = int(input("请输入你要下的位置(1-9):").strip())
if board[choice-1] == " ":
board[choice-1] = icon
else:
print("对不起,那个位置已经有棋子了!")
def is_victory(icon):
if (board[0] == icon and board[1] == icon and board[2] == icon) or \
(board[3] == icon and board[4] == icon and board[5] == icon) or \
(board[6] == icon and board[7] == icon and board[8] == icon) or \
(board[0] == icon and board[3] == icon and board[6] == icon) or \
(board[1] == icon and board[4] == icon and board[7] == icon) or \
(board[2] == icon and board[5] == icon and board[8] == icon) or \
(board[0] == icon and board[4] == icon and board[8] == icon) or \
(board[2] == icon and board[4] == icon and board[6] == icon):
return True
else:
return False
def is_draw():
if " " not in board:
return True
else:
return False
while True:
print_board()
player_move("X")
print_board()
if is_victory("X"):
print("恭喜你,玩家 1 获胜!")
break
elif is_draw():
print("平局!")
break
player_move("O")
if is_victory("O"):
print_board()
print("恭喜你,玩家 2 获胜!")
break
elif is_draw():
print("平局!")
break
```
3. 飞行棋游戏:
```python
import random
board = [
" ", " ", " ", " ", " ", " ", " ", " ", " ", " ",
" ", " ", " ", " ", " ", " ", " ", " ", " ", " ",
" ", " ", " ", " ", " ", " ", " ", " ", " ", " ",
" ", " ", " ", " ", " ", " ", " ", " ", " ", " ",
" ", " ", " ", " ", " ", " ", " ", " ", " ", " ",
" ", " ", " ", " ", " ", " ", " ", " ", " ", " ",
" ", " ", " ", " ", " ", " ", " ", " ", " ", " ",
" ", " ", " ", " ", " ", " ", " ", " ", " ", " ",
" ", " ", " ", " ", " ", " ", " ", " ", " ", " ",
" ", " ", " ", " ", " ", " ", " ", " ", " ", " ",
]
def print_board():
print("".join(["+" for i in range(31)]))
for i in range(10):
print("|" + "|".join(board[i*10:i*10+10]) + "|")
print("".join(["+" for i in range(31)]))
def move(player, steps):
pos = board.index(player)
board[pos] = " "
if pos+steps < 100:
board[pos+steps] = player
else:
board[99] = player
def roll_dice():
return random.randint(1, 6)
def get_winner():
if board[99] == "A":
return "玩家 A"
elif board[99] == "B":
return "玩家 B"
board[0] = "A"
board[99] = "B"
while True:
print_board()
input("按下 Enter 键掷骰子...")
steps = roll_dice()
print("你掷出了 {} 点".format(steps))
move("A", steps)
if board.index("A") == board.index("B"):
print_board()
print("玩家 B 被撞回了起点!")
board[0] = "B"
if board.index("A") == 99:
print_board()
print("恭喜你,你获胜了!")
break
input("按下 Enter 键掷骰子...")
steps = roll_dice()
print("你掷出了 {} 点".format(steps))
move("B", steps)
if board.index("A") == board.index("B"):
print_board()
print("玩家 A 被撞回了起点!")
board[0] = "A"
if board.index("B") == 99:
print_board()
print("恭喜你,玩家 B 获胜了!")
break
```
以上是三个简单的 Python 游戏示例,你可以根据需求进行修改和扩展。
阅读全文
相关推荐
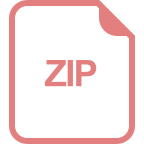
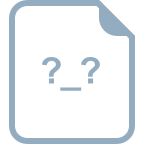
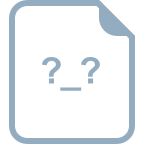
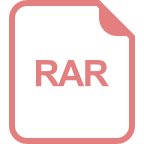
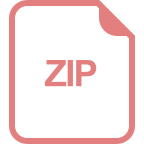
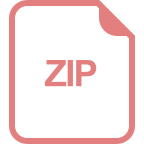
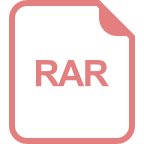
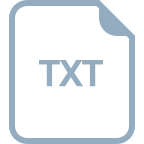
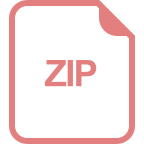
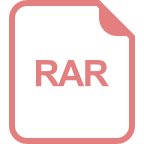
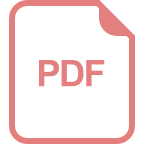
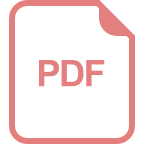
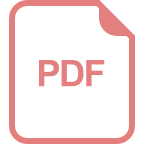