c语言写一棵查找二叉树
时间: 2023-10-26 10:12:33 浏览: 39
好的,以下是 C 语言实现的查找二叉树的代码:
```
#include<stdio.h>
#include<stdlib.h>
struct node {
int data;
struct node *left;
struct node *right;
};
struct node *insert(struct node *root, int x) {
if (root == NULL) {
struct node *temp = (struct node *)malloc(sizeof(struct node));
temp->data = x;
temp->left = NULL;
temp->right = NULL;
return temp;
}
if (x < root->data) {
root->left = insert(root->left, x);
} else {
root->right = insert(root->right, x);
}
return root;
}
void inorder(struct node *root) {
if (root == NULL) {
return;
}
inorder(root->left);
printf("%d ", root->data);
inorder(root->right);
}
int main() {
struct node *root = NULL;
int n, x;
scanf("%d", &n);
for (int i = 0; i < n; ++i) {
scanf("%d", &x);
root = insert(root, x);
}
inorder(root);
printf("\n");
return 0;
}
```
这是一个简单的查找二叉树的实现。先定义一个结构体表示树的一个节点,包括节点值和左右子节点指针。在插入时如果根节点为空则直接新建一个节点。如果要插入的值小于当前节点的值,则将其插入当前节点的左子树中;否则将其插入当前节点的右子树中。在遍历过程中按照中序遍历的顺序打印节点值。程序运行时输入节点个数和每个节点的值,输出中序遍历结果。
相关推荐
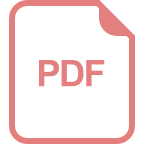
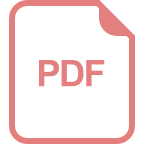
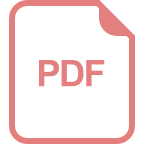
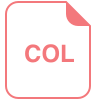
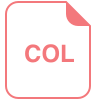
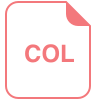
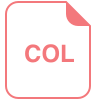
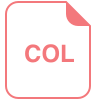









