使用java连接数据库编写一个员工信息管理系统的代码
时间: 2024-02-12 08:08:44 浏览: 30
好的,以下是一个简单的Java代码示例,用于连接到MySQL数据库并查询员工信息:
```java
import java.sql.*;
public class EmployeeManagementSystem {
static final String JDBC_DRIVER = "com.mysql.jdbc.Driver";
static final String DB_URL = "jdbc:mysql://localhost/employee_db";
static final String USER = "root";
static final String PASS = "password";
public static void main(String[] args) {
Connection conn = null;
Statement stmt = null;
try {
// 注册JDBC驱动程序
Class.forName(JDBC_DRIVER);
// 打开连接
System.out.println("连接数据库...");
conn = DriverManager.getConnection(DB_URL, USER, PASS);
// 执行查询
System.out.println("创建查询语句...");
stmt = conn.createStatement();
String sql = "SELECT id, name, age, gender FROM employees";
ResultSet rs = stmt.executeQuery(sql);
// 处理结果集
while (rs.next()) {
int id = rs.getInt("id");
String name = rs.getString("name");
int age = rs.getInt("age");
String gender = rs.getString("gender");
// 输出结果
System.out.print("ID: " + id);
System.out.print(", 姓名: " + name);
System.out.print(", 年龄: " + age);
System.out.println(", 性别: " + gender);
}
// 清理环境
rs.close();
stmt.close();
conn.close();
} catch (SQLException se) {
// 处理JDBC错误
se.printStackTrace();
} catch (Exception e) {
// 处理Class.forName错误
e.printStackTrace();
} finally {
// 关闭资源
try {
if (stmt != null) stmt.close();
} catch (SQLException se2) {
}
try {
if (conn != null) conn.close();
} catch (SQLException se) {
se.printStackTrace();
}
}
System.out.println("Goodbye!");
}
}
```
在这个示例中,我们首先定义了一些常量,包括JDBC驱动程序的名称、数据库的URL、用户名和密码。
然后,我们通过调用`Class.forName()`方法来注册JDBC驱动程序。接下来,我们使用`DriverManager.getConnection()`方法来打开数据库连接,并创建一个`Statement`对象来执行SQL查询。
在这个示例中,我们查询了一个名为`employees`的表中的所有员工信息,并使用`ResultSet`对象来处理查询结果。最后,我们在`finally`块中关闭了所有的资源。
当然,这只是一个简单的示例。在实际编写员工信息管理系统时,您需要更多地了解JDBC API以及如何使用它来实现增加、更新和删除等操作。
相关推荐
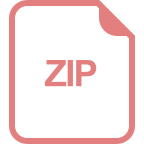
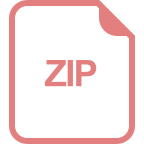
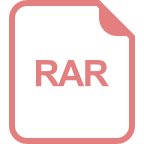














