写一个stm32c8t6按键控制程序,PB14输出按键一控制PA8的蜂鸣器,PB15输出按键二控制PA15的LED
时间: 2024-05-15 20:19:54 浏览: 103
以下是一个简单的按键控制程序,实现了PB14控制PA8蜂鸣器和PB15控制PA15 LED的功能。需要注意的是,为了避免按键的抖动,需要加入软件消抖机制。
```
#include "stm32f1xx.h"
// 定义按键GPIO端口和引脚
#define KEY1_GPIO_Port GPIOB
#define KEY1_Pin GPIO_PIN_14
#define KEY2_GPIO_Port GPIOB
#define KEY2_Pin GPIO_PIN_15
// 定义蜂鸣器和LED GPIO端口和引脚
#define BEEP_GPIO_Port GPIOA
#define BEEP_Pin GPIO_PIN_8
#define LED_GPIO_Port GPIOA
#define LED_Pin GPIO_PIN_15
// 软件消抖函数
uint8_t debounce(GPIO_TypeDef* GPIOx, uint16_t GPIO_Pin)
{
uint8_t count = 0;
uint8_t last_state = HAL_GPIO_ReadPin(GPIOx, GPIO_Pin);
while (count < 5)
{
if (HAL_GPIO_ReadPin(GPIOx, GPIO_Pin) != last_state)
{
count = 0;
last_state = HAL_GPIO_ReadPin(GPIOx, GPIO_Pin);
}
else
{
count++;
}
HAL_Delay(1);
}
return last_state;
}
int main(void)
{
// 初始化GPIO
HAL_Init();
__HAL_RCC_GPIOA_CLK_ENABLE();
__HAL_RCC_GPIOB_CLK_ENABLE();
GPIO_InitTypeDef GPIO_InitStruct = {0};
GPIO_InitStruct.Pin = BEEP_Pin | LED_Pin;
GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP;
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW;
HAL_GPIO_Init(BEEP_GPIO_Port, &GPIO_InitStruct);
HAL_GPIO_Init(LED_GPIO_Port, &GPIO_InitStruct);
GPIO_InitStruct.Pin = KEY1_Pin | KEY2_Pin;
GPIO_InitStruct.Mode = GPIO_MODE_INPUT;
GPIO_InitStruct.Pull = GPIO_PULLUP;
HAL_GPIO_Init(KEY1_GPIO_Port, &GPIO_InitStruct);
HAL_GPIO_Init(KEY2_GPIO_Port, &GPIO_InitStruct);
while (1)
{
// 检测按键一
if (debounce(KEY1_GPIO_Port, KEY1_Pin) == GPIO_PIN_RESET)
{
HAL_GPIO_WritePin(BEEP_GPIO_Port, BEEP_Pin, GPIO_PIN_SET);
HAL_Delay(50);
HAL_GPIO_WritePin(BEEP_GPIO_Port, BEEP_Pin, GPIO_PIN_RESET);
}
// 检测按键二
if (debounce(KEY2_GPIO_Port, KEY2_Pin) == GPIO_PIN_RESET)
{
HAL_GPIO_WritePin(LED_GPIO_Port, LED_Pin, GPIO_PIN_SET);
HAL_Delay(500);
HAL_GPIO_WritePin(LED_GPIO_Port, LED_Pin, GPIO_PIN_RESET);
}
}
}
```
阅读全文
相关推荐
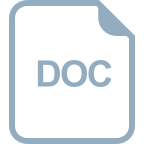
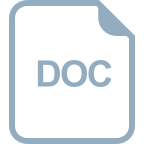
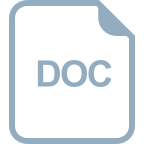
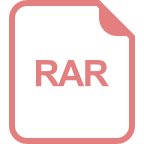
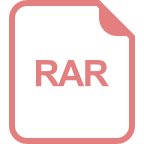













