vector<pair<int, string>> attribute;
时间: 2023-12-17 11:05:44 浏览: 19
This is a declaration of a vector container named "attribute" that can store pairs of integer and string data types. Each element in the vector will be a pair consisting of an integer value and a corresponding string value.
相关推荐
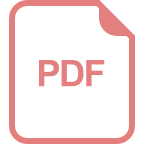
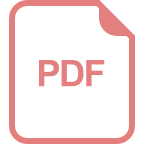
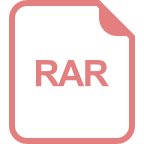
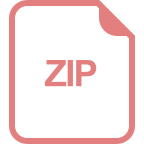
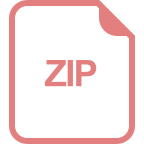
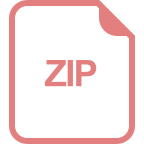
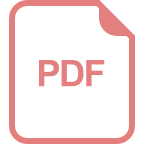