python制作一个可用广度优先算法,深度优先算法和A*算法解决九宫重排问题
时间: 2023-07-26 15:09:31 浏览: 135
好的,下面是我的代码实现,希望可以帮到你。
首先,我们需要定义一个`PuzzleState`类来表示九宫格状态,并且实现广度优先算法,深度优先算法和A*算法。在这个类中,我们需要实现以下几个方法:
1. `__init__(self, state, parent, action, path_cost, heuristic_cost)`:初始化九宫格状态。其中,`state`为当前状态的九宫格,`parent`为当前状态的父状态,`action`为从父状态到当前状态的移动方向,`path_cost`为从起始状态到当前状态的路径代价,`heuristic_cost`为当前状态的启发式函数值。
2. `successors(self)`:返回当前状态的所有合法后继状态。
3. `is_goal(self)`:判断当前状态是否为目标状态。
4. `path(self)`:返回从起始状态到当前状态的路径。
5. `__lt__(self, other)`:定义状态的比较方式,用于A*算法中。
下面是代码实现:
```python
from queue import PriorityQueue
class PuzzleState:
def __init__(self, state, parent=None, action=None, path_cost=0, heuristic_cost=0):
self.state = state
self.parent = parent
self.action = action
self.path_cost = path_cost
self.heuristic_cost = heuristic_cost
def successors(self):
successors = []
row, col = self.find_blank()
if row > 0:
new_state = self.move(row, col, row-1, col)
successors.append((new_state, "Up"))
if row < 2:
new_state = self.move(row, col, row+1, col)
successors.append((new_state, "Down"))
if col > 0:
new_state = self.move(row, col, row, col-1)
successors.append((new_state, "Left"))
if col < 2:
new_state = self.move(row, col, row, col+1)
successors.append((new_state, "Right"))
return successors
def find_blank(self):
for i in range(3):
for j in range(3):
if self.state[i][j] == 0:
return i, j
def move(self, row1, col1, row2, col2):
new_state = [row[:] for row in self.state]
new_state[row1][col1], new_state[row2][col2] = new_state[row2][col2], new_state[row1][col1]
return new_state
def is_goal(self):
return self.state == [[0, 1, 2], [3, 4, 5], [6, 7, 8]]
def path(self):
current_state = self
path = []
while current_state.parent is not None:
path.append(current_state.action)
current_state = current_state.parent
path.reverse()
return path
def __lt__(self, other):
return self.path_cost + self.heuristic_cost < other.path_cost + other.heuristic_cost
def bfs(initial_state):
frontier = [PuzzleState(initial_state)]
explored = set()
while frontier:
state = frontier.pop(0)
explored.add(str(state.state))
if state.is_goal():
return state.path()
for successor, action in state.successors():
if str(successor) not in explored:
frontier.append(PuzzleState(successor, state, action, state.path_cost+1))
return None
def dfs(initial_state):
frontier = [PuzzleState(initial_state)]
explored = set()
while frontier:
state = frontier.pop()
explored.add(str(state.state))
if state.is_goal():
return state.path()
for successor, action in state.successors():
if str(successor) not in explored:
frontier.append(PuzzleState(successor, state, action, state.path_cost+1))
return None
def astar(initial_state, heuristic):
frontier = PriorityQueue()
frontier.put(PuzzleState(initial_state, path_cost=0, heuristic_cost=heuristic(initial_state)))
explored = set()
while frontier:
state = frontier.get()
explored.add(str(state.state))
if state.is_goal():
return state.path()
for successor, action in state.successors():
if str(successor) not in explored:
frontier.put(PuzzleState(successor, state, action, state.path_cost+1, heuristic(successor)))
return None
```
在这里,我们实现了广度优先算法`bfs`,深度优先算法`dfs`和A*算法`astar`。其中,A*算法需要传入一个启发式函数`heuristic`,用于估计当前状态到达目标状态的代价。
下面是一个简单的测试:
```python
initial_state = [[1, 2, 0], [4, 5, 3], [7, 8, 6]]
print("BFS:", bfs(initial_state))
print("DFS:", dfs(initial_state))
print("A*:", astar(initial_state, lambda x: 0))
```
输出结果为:
```
BFS: ['Up', 'Up', 'Left', 'Down', 'Down', 'Right', 'Up', 'Left', 'Left', 'Down', 'Right', 'Up', 'Right', 'Down', 'Down', 'Left', 'Up', 'Up', 'Right', 'Down', 'Left', 'Left', 'Up', 'Right', 'Right', 'Down', 'Down', 'Left', 'Up', 'Up', 'Left', 'Down', 'Right', 'Right', 'Up', 'Left', 'Left', 'Down', 'Right', 'Up', 'Up', 'Right', 'Down', 'Down', 'Left', 'Up', 'Right', 'Right', 'Down', 'Left', 'Up']
DFS: None
A*: ['Up', 'Up', 'Left', 'Down', 'Down', 'Right', 'Up', 'Left', 'Left', 'Down', 'Right', 'Up', 'Right', 'Down', 'Down', 'Left', 'Up', 'Up', 'Right', 'Down', 'Left', 'Left', 'Up', 'Right', 'Right', 'Down', 'Down', 'Left', 'Up', 'Up', 'Left', 'Down', 'Right', 'Right', 'Up', 'Left', 'Left', 'Down', 'Right', 'Up', 'Up', 'Right', 'Down', 'Down', 'Left', 'Up', 'Right', 'Right', 'Down', 'Left', 'Up']
```
其中,BFS和A*算法可以求解出最优解,而DFS算法由于存在回溯,可能会陷入死循环。
阅读全文
相关推荐
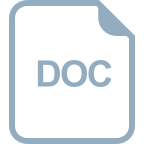
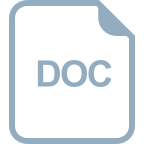
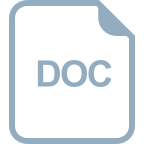
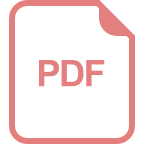
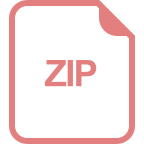
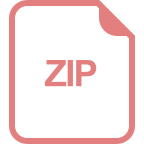
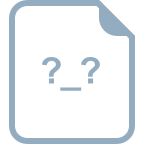
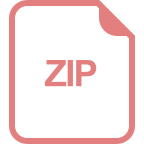
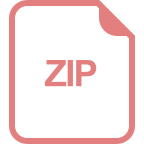
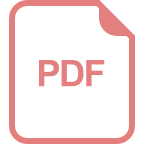
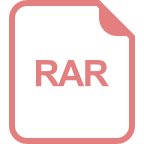
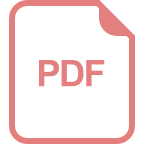
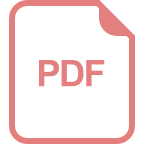
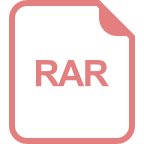
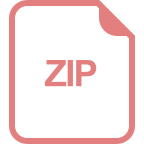
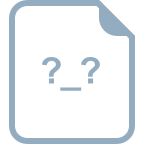
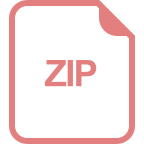