从键盘任意输入一个字符,编程判断该字符是数字字符、英文字母、空格还是其他字符。 **输入格式要求:提示信息:"press a key and then press enter:" 用 getchar()输入
时间: 2023-05-31 15:20:16 浏览: 155
### 回答1:
该问题需要编写一个程序来判断用户输入的字符是数字字符、英文字母、空格还是其他字符。这个程序需要提示用户输入一个字符,然后使用getchar()函数来获取用户的输入。然后,程序需要使用一个if语句的链来测试输入字符的种类,并输出结果。
具体实现代码如下:
```c
#include <stdio.h>
int main() {
char ch;
printf("请输入一个字符:");
ch = getchar();
if (ch >= '0' && ch <= '9') {
printf("您输入的是数字字符。\n");
} else if ((ch >= 'a' && ch <= 'z') || (ch >= 'A' && ch <= 'Z')) {
printf("您输入的是英文字母。\n");
} else if (ch == ' ') {
printf("您输入的是空格。\n");
} else {
printf("您输入的是其他字符。\n");
}
return 0;
}
```
运行程序后,会先提示用户输入一个字符。用户输入完一个字符,按下回车键后,程序会根据用户输入的字符输出相应的结果。如果输入的是数字字符,就会输出“您输入的是数字字符。”,如果输入的是英文字母,就会输出“您输入的是英文字母。”,如果输入的是空格,则会输出“您输入的是空格。”,否则,就会输出“您输入的是其他字符。”。
例如,当用户输入字符'A'时,程序会输出“您输入的是英文字母。”
### 回答2:
要实现这个功能,需要使用C语言中的字符函数库中的isalpha()、isdigit()和isspace()函数分别来判断输入字符是否为英文字母、数字字符和空格。同时,我们需要判断该字符是否为其他字符,需要对输入的字符ASCII码进行比较。
实现方法如下:
1. 使用getchar()函数获取用户输入的字符:
```c
printf("press a key and then press enter: ");
char ch = getchar();
```
2. 使用isalpha()函数判断输入字符是否为英文字母:
```c
if (isalpha(ch)) {
printf("%c is an alphabetic character.\n", ch);
}
```
3. 使用isdigit()函数判断输入字符是否为数字字符:
```c
else if (isdigit(ch)) {
printf("%c is a digit character.\n", ch);
}
```
4. 使用isspace()函数判断输入字符是否为空格:
```c
else if (isspace(ch)) {
printf("%c is a space character.\n", ch);
}
```
5. 对于其他字符,通过ASCII码范围进行判断,并在最后加上默认情况:
```c
else {
if (ch >= 32 && ch <= 126) {
printf("%c is a special character.\n", ch);
} else {
printf("%c is not a printable character.\n", ch);
}
}
```
完整代码如下:
```
#include <stdio.h>
#include <ctype.h>
int main() {
printf("press a key and then press enter: ");
char ch = getchar();
if (isalpha(ch)) {
printf("%c is an alphabetic character.\n", ch);
} else if (isdigit(ch)) {
printf("%c is a digit character.\n", ch);
} else if (isspace(ch)) {
printf("%c is a space character.\n", ch);
} else {
if (ch >= 32 && ch <= 126) {
printf("%c is a special character.\n", ch);
} else {
printf("%c is not a printable character.\n", ch);
}
}
return 0;
}
```
运行程序后,屏幕会提示用户输入一个字符,用户输入后按下回车键,程序会判断该字符是数字字符、英文字母、空格还是其他字符,并输出相应的提示信息。
### 回答3:
首先,我们需要了解ASCII码表,它是一种用来表示字符的编码规范,其中数字字符、英文字母、空格等都有自己的编码。数字字符的编码范围是48~57,英文字母的编码范围是65~90和97~122,空格的编码是32,其他字符的编码就是除了上述范围之外的所有字符。
那么,我们可以根据输入的字符的ASCII码值来判断它是什么类型的字符。具体实现可以用getchar()函数来获取用户输入的字符,然后用if语句进行判断。代码如下:
```
#include<stdio.h>
int main()
{
char c;
printf("press a key and then press enter:\n");
c = getchar(); //获取用户输入的字符
if(c >= '0' && c <= '9') //判断是否为数字字符
printf("This is a digit.\n");
else if((c >= 'a' && c <= 'z')||(c >= 'A' && c <= 'Z')) //判断是否为英文字母
printf("This is a letter.\n");
else if(c == ' ') //判断是否为空格
printf("This is a space.\n");
else //其他情况
printf("This is another character.\n");
return 0;
}
```
在上面的代码中,我们通过if语句判断输入的字符类型,并输出相应的提示信息。注意,我们用单引号括起来的字符,相当于表示该字符的ASCII码值。同时,我们还需要注意格式要求,即在提示信息后面要加上一个换行符,使输出看起来更加美观。
这样,我们就可以通过一个简单的程序,判断用户输入的字符类型,为后续的操作提供便利。
相关推荐
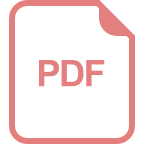
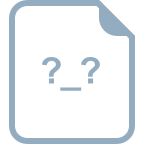
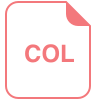
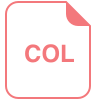
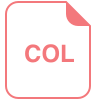
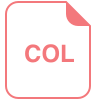
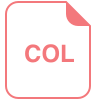









