疏散模型python源代码
时间: 2023-05-13 15:02:07 浏览: 229
疏散模型是模拟人群在紧急情况下从场地中疏散出来的过程的一种模型。下面是一个基于Python的简单疏散模型代码:
```python
import numpy as np
import matplotlib.pyplot as plt
import random
# 假设场地中有300个人,场地长宽为50米,总时间为40秒
N = 300
L = 50
T = 40
# 随机初始化每个人的位置和速度
x = np.random.rand(N)*L
y = np.random.rand(N)*L
vx = np.zeros(N)
vy = np.zeros(N)
# 安放出口位置
exit_x = L/2 + 2
exit_y = 0
# 模拟运动过程
for t in range(T*100):
# 计算人与人之间的距离
distance = np.sqrt((x-x[:,np.newaxis])**2 + (y-y[:,np.newaxis])**2)
# 排除自身以及距离过远的人
distance[np.arange(N),np.arange(N)] = np.inf
distance[distance > 3] = np.inf
# 找到每个人视野内最近的人
nearest = np.argmin(distance, axis=0)
# 计算每个人的运动方向
dx = (x - x[nearest]) / distance[nearest]
dy = (y - y[nearest]) / distance[nearest]
# 距离出口较近的人获得更大的速度
to_exit = np.sqrt((x-exit_x)**2 + (y-exit_y)**2)
factor = 1 - np.clip(to_exit/10, 0, 1)
# 计算速度
vx += dx * factor
vy += dy * factor
# 限制速度不超过2
v = np.sqrt(vx**2 + vy**2)
v[v > 2] = 2
vx *= v / (np.sqrt(vx**2 + vy**2) + 1e-10)
vy *= v / (np.sqrt(vx**2 + vy**2) + 1e-10)
# 更新位置
x += vx
y += vy
# 边界处理
x[x < 0] = 0
y[y < 0] = 0
x[x > L] = L
y[y > L] = L
# 到达出口的人停留并退出
arrive = np.where(to_exit < 2)[0]
vx[arrive] = 0
vy[arrive] = 0
x[arrive] = exit_x
y[arrive] = exit_y - 1
N -= len(arrive)
# 如果所有人都已经疏散完成,则终止模拟
if N == 0:
break
# 绘图
plt.figure(figsize=(8, 8))
plt.scatter(x, y)
plt.scatter(exit_x, exit_y, color='r')
plt.xlim(0, L)
plt.ylim(0, L)
plt.title('Evacuation Simulation')
plt.xlabel('X Position')
plt.ylabel('Y Position')
plt.show()
```
该代码通过随机初始化每个人的位置和速度,并根据人与人之间的距离计算运动方向和速度,模拟人群在场地中的运动过程。同时,在距离出口较近的人获得更大的速度的条件下,到达出口的人停留并退出,直到所有人都已经疏散完成。最终通过Matplotlib将疏散过程可视化出来。
相关推荐
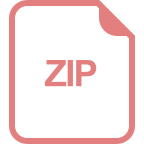














