用pathon写一个商品管理系统
时间: 2024-03-16 08:41:39 浏览: 72
好的,以下是一个简单的商品管理系统的Python代码示例:
```python
class Product:
def __init__(self, name, price, quantity):
self.name = name
self.price = price
self.quantity = quantity
class ProductManager:
def __init__(self):
self.products = []
def add_product(self, product):
self.products.append(product)
print(f"商品 {product.name} 添加成功!")
def remove_product(self, product):
if product in self.products:
self.products.remove(product)
print(f"商品 {product.name} 移除成功!")
else:
print(f"商品 {product.name} 不存在!")
def update_product(self, product, new_name=None, new_price=None, new_quantity=None):
if product in self.products:
if new_name:
product.name = new_name
if new_price:
product.price = new_price
if new_quantity:
product.quantity = new_quantity
print(f"商品 {product.name} 更新成功!")
else:
print(f"商品 {product.name} 不存在!")
def print_products(self):
print("所有商品信息如下:")
for product in self.products:
print(f"名称:{product.name},价格:{product.price},数量:{product.quantity}")
# 创建商品管理器实例
manager = ProductManager()
# 添加商品
p1 = Product("苹果", 5.0, 10)
manager.add_product(p1)
p2 = Product("香蕉", 3.0, 20)
manager.add_product(p2)
# 更新商品
manager.update_product(p1, new_name="红苹果")
manager.update_product(p2, new_price=4.0)
# 打印所有商品信息
manager.print_products()
# 移除商品
manager.remove_product(p1)
# 打印所有商品信息
manager.print_products()
```
这个商品管理系统包含了一个 `Product` 类来表示商品,一个 `ProductManager` 类来管理所有商品。你可以通过调用 `ProductManager` 类中的方法来添加、移除、更新和打印商品信息。根据需要,你可以扩展这个商品管理系统,添加更多的功能,例如根据价格或数量查询商品,或者将商品信息保存到文件中等。
阅读全文
相关推荐
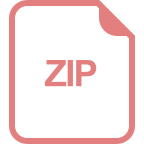

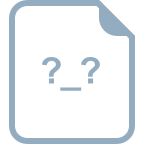
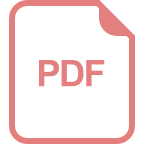
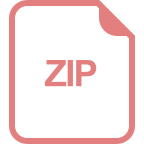
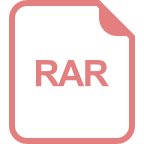
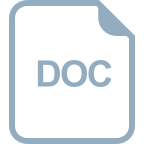
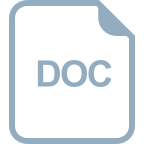




