Create a HistoryPanel class that extends JPanel. The constructor of HistoryPanel takes as argument a model object of type Library, which you need to store in some private instance variable.
时间: 2023-12-10 22:41:48 浏览: 121
Sure, here's an example implementation of the `HistoryPanel` class:
```java
import javax.swing.*;
import java.util.List;
public class HistoryPanel extends JPanel {
private final Library library;
private final JTextArea textArea;
public HistoryPanel(Library library) {
this.library = library;
// set layout and other properties of the panel
setLayout(new BoxLayout(this, BoxLayout.Y_AXIS));
setBorder(BorderFactory.createTitledBorder("History"));
// create text area to display history
textArea = new JTextArea(20, 30);
textArea.setEditable(false);
// add text area to a scroll pane and add to the panel
JScrollPane scrollPane = new JScrollPane(textArea);
add(scrollPane);
// display initial history
updateHistory();
}
private void updateHistory() {
List<Book> history = library.getHistory();
StringBuilder sb = new StringBuilder();
for (Book book : history) {
sb.append(book.getTitle()).append(" by ").append(book.getAuthor()).append("\n");
}
textArea.setText(sb.toString());
}
}
```
In this implementation, the `HistoryPanel` class extends `JPanel` and has two instance variables: `library` and `textArea`. The constructor takes a `Library` object as an argument, which is stored in the `library` instance variable. The `textArea` instance variable is a `JTextArea` used to display the history of checked-out books.
The `updateHistory()` method is called to update the text displayed in the `textArea`. It retrieves the list of checked-out books from the `library` object, formats the titles and authors of the books into a single string, and sets that string as the text of the `textArea`.
The `HistoryPanel` constructor also sets the layout of the panel, creates a titled border, adds the `textArea` to a scroll pane, and adds the scroll pane to the panel. Finally, it calls `updateHistory()` to display the initial history.
阅读全文
相关推荐













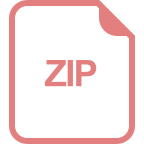



