已知椭圆的两焦点坐标,及长轴短轴长度,用python绘制该椭圆
时间: 2024-02-25 17:53:22 浏览: 186
你可以使用 Python 中的 matplotlib 库来绘制椭圆,具体步骤如下:
1. 导入 matplotlib 库:
```python
import matplotlib.pyplot as plt
```
2. 计算椭圆的中心坐标和半轴长度:
```python
x0 = (x1 + x2) / 2
y0 = (y1 + y2) / 2
a = abs(x2 - x1) / 2
b = abs(y2 - y1) / 2
```
其中,(x1, y1) 和 (x2, y2) 分别表示椭圆的两个焦点坐标。
3. 绘制椭圆:
```python
theta = np.arange(0, 2*np.pi, 0.01)
x = x0 + a*np.cos(theta)
y = y0 + b*np.sin(theta)
plt.plot(x, y)
```
其中,np.arange(0, 2*np.pi, 0.01) 表示从 0 到 2π(即一周)每隔 0.01 弧度取一个点,x 和 y 分别表示椭圆上每个点的横纵坐标,plt.plot(x, y) 表示将这些点连接起来绘制椭圆。
4. 显示图像:
```python
plt.axis('equal') # 设置横纵坐标轴的比例为相等
plt.show()
```
完整的代码示例:
```python
import numpy as np
import matplotlib.pyplot as plt
# 定义椭圆的两个焦点坐标和长轴短轴长度
x1, y1 = -2, 0
x2, y2 = 2, 0
a, b = 3, 2
# 计算椭圆的中心坐标和半轴长度
x0 = (x1 + x2) / 2
y0 = (y1 + y2) / 2
a = abs(x2 - x1) / 2
b = abs(y2 - y1) / 2
# 绘制椭圆
theta = np.arange(0, 2*np.pi, 0.01)
x = x0 + a*np.cos(theta)
y = y0 + b*np.sin(theta)
plt.plot(x, y)
# 显示图像
plt.axis('equal')
plt.show()
```
运行以上代码,即可绘制出该椭圆。
阅读全文
相关推荐
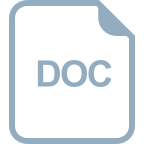
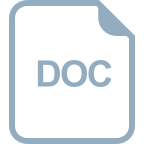
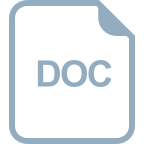









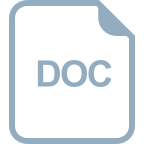
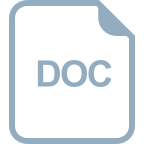
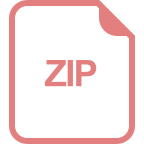
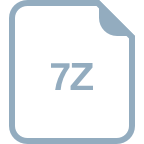