请写一个输入ABCD四个字母入栈(不一定是顺序入栈),有24种不同出栈顺序的代码,java实现
时间: 2024-03-03 12:52:40 浏览: 49
好的,下面是一个可能的实现:
```java
import java.util.*;
public class StackPermutations {
public static void main(String[] args) {
char[] input = {'A', 'B', 'C', 'D'};
ArrayList<ArrayList<Character>> permutations = generatePermutations(input);
for (ArrayList<Character> permutation : permutations) {
System.out.println(permutation);
}
}
public static ArrayList<ArrayList<Character>> generatePermutations(char[] input) {
ArrayList<ArrayList<Character>> permutations = new ArrayList<>();
Stack<Character> stack = new Stack<>();
generatePermutationsHelper(input, 0, stack, new ArrayList<>(), permutations);
return permutations;
}
private static void generatePermutationsHelper(char[] input, int index, Stack<Character> stack,
ArrayList<Character> output, ArrayList<ArrayList<Character>> permutations) {
if (index == input.length && stack.isEmpty()) {
permutations.add(new ArrayList<>(output));
return;
}
if (!stack.isEmpty()) {
output.add(stack.pop());
generatePermutationsHelper(input, index, stack, output, permutations);
stack.push(output.remove(output.size() - 1));
}
if (index < input.length) {
stack.push(input[index]);
generatePermutationsHelper(input, index + 1, stack, output, permutations);
stack.pop();
}
}
}
```
这个程序的思路是,首先将输入的字母依次入栈,然后通过递归生成不同的出栈顺序。在递归过程中,如果栈非空,则可以弹出栈顶元素并将其加入输出序列中;如果还有字母没有入栈,则可以将下一个字母入栈。最终,当所有字母都入栈且栈为空时,就生成了一种出栈顺序。程序使用了两个辅助数据结构:一个栈用于模拟入栈和出栈操作,一个列表用于存储当前的输出序列。程序的输出是一个列表,其中每个元素表示一种不同的出栈顺序。
阅读全文
相关推荐




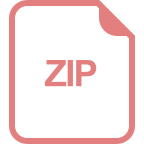
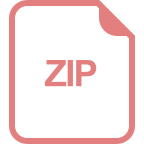
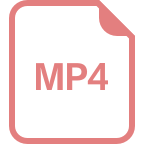
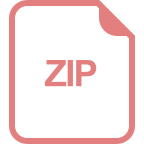
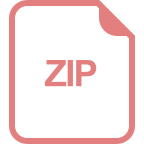
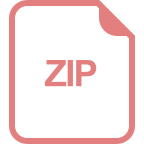
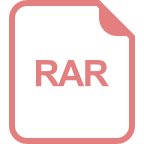
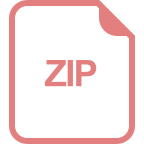