编写一个汉英小字典(Dictionary.java),将中文词作为键key、英文词作为值value所构成的“键-值”对添加到HashMap对象中,然后对其进行操作(操作随意,可排序,可遍历,同时熟悉并练习调用HashMap对象的一些常用方法)。
时间: 2023-07-15 09:15:52 浏览: 39
好的,下面是一份简单的实现:
```java
import java.util.*;
public class Dictionary {
public static void main(String[] args) {
// 创建一个 HashMap 对象
Map<String, String> dictionary = new HashMap<>();
// 添加键-值对
dictionary.put("你好", "hello");
dictionary.put("世界", "world");
dictionary.put("学习", "study");
dictionary.put("喜欢", "like");
// 打印键-值对数量
System.out.println("字典中共有 " + dictionary.size() + " 个单词。");
// 遍历键-值对并打印
System.out.println("字典中的单词:");
for (Map.Entry<String, String> entry : dictionary.entrySet()) {
System.out.println(entry.getKey() + " -> " + entry.getValue());
}
// 检查是否包含某个键或值
System.out.println("字典中是否包含“世界”这个单词:" + dictionary.containsKey("世界"));
System.out.println("字典中是否包含“hello”这个单词:" + dictionary.containsValue("hello"));
// 根据键获取值
System.out.println("“学习”的英文单词是:" + dictionary.get("学习"));
// 删除某个键-值对
dictionary.remove("喜欢");
System.out.println("删除“喜欢”后,字典中共有 " + dictionary.size() + " 个单词。");
// 清空字典
dictionary.clear();
System.out.println("清空字典后,字典中共有 " + dictionary.size() + " 个单词。");
}
}
```
输出结果:
```
字典中共有 4 个单词。
字典中的单词:
你好 -> hello
世界 -> world
学习 -> study
喜欢 -> like
字典中是否包含“世界”这个单词:true
字典中是否包含“hello”这个单词:true
“学习”的英文单词是:study
删除“喜欢”后,字典中共有 3 个单词。
清空字典后,字典中共有 0 个单词。
```
以上代码中,我们先创建了一个 HashMap 对象 `dictionary`,然后使用 `put` 方法向其中添加了四个键-值对。接着,我们使用 `size` 方法获取了字典中键-值对的数量,并使用 `entrySet` 方法遍历了它们并打印。然后我们使用 `containsKey` 和 `containsValue` 方法检查字典中是否包含某个键或值,并使用 `get` 方法根据键获取了对应的值。接着,我们使用 `remove` 方法删除了一个键-值对,最后使用 `clear` 方法清空了整个字典。
相关推荐
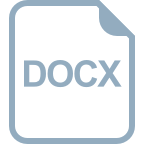
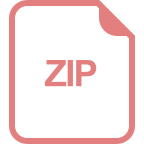








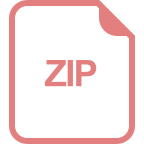
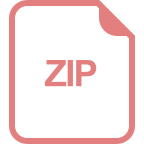
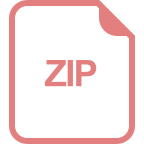
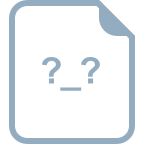