uniapp vue3 纵向瀑布流使用计算高度的方法实现
时间: 2023-12-20 15:22:59 浏览: 32
在Vue3中实现纵向瀑布流布局,也可以使用计算高度的方法。具体步骤如下:
1. 在组件的 `onLoad` 生命周期中获取屏幕宽度,计算每个 item 的宽度;
2. 在数据加载完成后,遍历数据并计算每个 item 的高度,可以使用 `uni.getImageInfo` 方法获取图片信息,进而计算图片的高度;
3. 将每个 item 的高度保存到数据中,并根据列数进行分组,每一组的高度即为该组中所有 item 的高度之和;
4. 在模板中使用 `v-for` 遍历每一组,设置 `display: flex` 和 `flex-direction: column`,实现纵向瀑布流布局;
5. 使用 `style` 绑定每个 item 的高度和宽度,以及 `margin` 或 `padding` 等样式,实现美观的布局效果。
下面是一个简单的示例代码:
```html
<template>
<div class="container">
<div
class="item"
v-for="(group, index) in groupList"
:key="index"
:style="'height: ' + group.height + 'px; width: ' + itemWidth + 'px'"
>
<img
class="img"
:src="item.image"
:style="'height: ' + item.height + 'px'"
mode="aspectFill"
/>
</div>
</div>
</template>
<script>
import { ref, onMounted } from 'vue';
export default {
setup() {
const dataList = [
{ image: 'url1' },
{ image: 'url2' },
{ image: 'url3' },
// ...
];
const columnCount = 2;
const itemWidth = ref(0);
const groupList = ref([]);
const onLoad = () => {
const { windowWidth } = uni.getSystemInfoSync();
itemWidth.value = windowWidth / columnCount;
};
const calculateHeight = () => {
const tempDataList = [...dataList];
const tempGroupList = Array.from({ length: columnCount }, () => ({
height: 0,
items: []
}));
while (tempDataList.length) {
const minGroupIndex = tempGroupList.reduce((pre, cur, index) => {
if (cur.height < tempGroupList[pre].height) {
return index;
}
return pre;
}, 0);
const item = tempDataList.shift();
const { width } = uni.getImageInfoSync({
src: item.image
});
const height = width * 300 / 200;
item.height = height;
tempGroupList[minGroupIndex].height += height;
tempGroupList[minGroupIndex].items.push(item);
}
groupList.value = tempGroupList;
};
onMounted(() => {
calculateHeight();
});
return {
itemWidth,
groupList,
onLoad
};
}
};
</script>
<style>
.container {
display: flex;
flex-wrap: wrap;
}
.item {
margin: 10px;
background-color: #eee;
overflow: hidden;
display: flex;
flex-direction: column;
}
.img {
width: 100%;
}
</style>
```
需要注意的是,在Vue3中,我们使用了 `ref` 和 `setup` 方法来实现组件逻辑。同时,由于 `uni.getImageInfo` 方法是异步的,所以我们需要在 `onMounted` 生命周期中调用 `calculateHeight` 方法来确保数据加载完成后再计算高度。
相关推荐
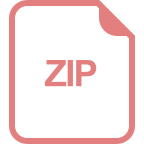
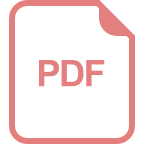
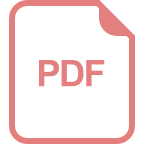














