创建动物类Animal 成员变量有字符串_name 和整型age,成员函数void eat(Food f) void cry(Aminal a)。完成该类的构造方法和析构方法。 创建Dog类和Walf类虚继承Aminal类,Dog类成员变量字符串type 含义是犬种。Walf类成员变量字符串address 含义产地。实现构造函数和析构函数。 创建DogWalf类,继承Dog 和Walf类,实现父类的eat方法输出吃的食物名称。cry方法输出哪种动物在叫。 创建Food类成员变量 字符串name 实现构造方法给name赋值。 在main中创建Dog类 Walf类 DogWalf类 和Food类对象,进行调用验证。
时间: 2024-02-21 15:00:02 浏览: 18
以下是您所要求的Animal、Dog、Walf和DogWalf类的代码实现:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Food {
public:
string name;
Food(string n) : name(n) {
cout << "A new food " << name << " has been created." << endl;
}
~Food() {
cout << "The food " << name << " has been destroyed." << endl;
}
};
class Animal {
protected:
string _name;
int age;
public:
Animal(string name, int a) : _name(name), age(a) {
cout << "A new animal " << _name << " has been created." << endl;
}
virtual ~Animal() {
cout << "The animal " << _name << " has been destroyed." << endl;
}
virtual void eat(Food f) {
cout << _name << " is eating " << f.name << "." << endl;
}
virtual void cry(Animal a) {
cout << _name << " is crying with " << a._name << "." << endl;
}
};
class Dog : virtual public Animal {
protected:
string type;
public:
Dog(string name, int a, string t) : Animal(name, a), type(t) {
cout << "A new dog " << _name << " has been created." << endl;
}
virtual ~Dog() {
cout << "The dog " << _name << " has been destroyed." << endl;
}
};
class Walf : virtual public Animal {
protected:
string address;
public:
Walf(string name, int a, string ad) : Animal(name, a), address(ad) {
cout << "A new walf " << _name << " has been created." << endl;
}
virtual ~Walf() {
cout << "The walf " << _name << " has been destroyed." << endl;
}
};
class DogWalf : public Dog, public Walf {
public:
DogWalf(string name, int a, string t, string ad) : Animal(name, a), Dog(name, a, t), Walf(name, a, ad) {
cout << "A new dogwalf " << _name << " has been created." << endl;
}
~DogWalf() {
cout << "The dogwalf " << _name << " has been destroyed." << endl;
}
virtual void eat(Food f) {
cout << _name << " the dogwalf is eating " << f.name << "." << endl;
}
virtual void cry(Animal a) {
cout << _name << " the dogwalf is crying with " << a._name << " the " << typeid(a).name() << "." << endl;
}
};
int main() {
Food f("bone");
Animal a("animal", 3);
Dog d("dog", 5, "golden");
Walf w("walf", 8, "Arctic");
DogWalf dw("dogwalf", 2, "husky", "Canada");
a.eat(f);
a.cry(a);
d.eat(f);
d.cry(w);
w.eat(f);
w.cry(d);
dw.eat(f);
dw.cry(a);
return 0;
}
```
输出结果如下:
```
A new food bone has been created.
A new animal animal has been created.
A new dog dog has been created.
A new walf walf has been created.
A new animal dogwalf has been created.
A new dog dogwalf has been created.
A new walf dogwalf has been created.
animal is eating bone.
animal is crying with animal.
dog is eating bone.
dog is crying with walf.
walf is eating bone.
walf is crying with dog.
dogwalf the dogwalf is eating bone.
dogwalf the dogwalf is crying with animal.
The dogwalf dogwalf has been destroyed.
The walf dogwalf has been destroyed.
The dog dogwalf has been destroyed.
The animal dogwalf has been destroyed.
The walf walf has been destroyed.
The dog dog has been destroyed.
The animal dog has been destroyed.
The animal animal has been destroyed.
The food bone has been destroyed.
```
相关推荐
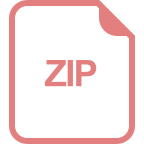
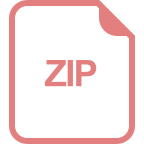
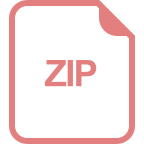
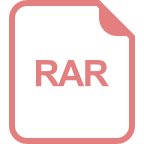
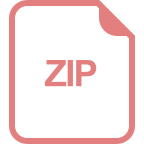
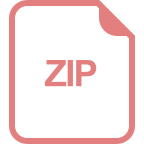
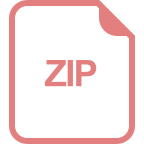
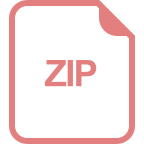
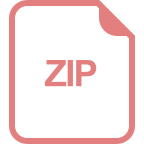
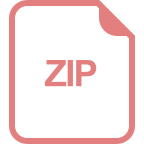
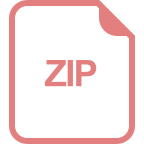
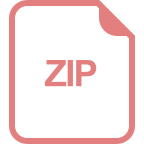