用c++实现信息加密程序,使用DES算法,编写代码
时间: 2023-11-20 08:07:14 浏览: 77
好的,以下是使用C++实现信息加密程序,使用DES算法的示例代码:
```c++
#include <iostream>
#include <cstring>
#include <openssl/des.h>
using namespace std;
// 加密函数
void encrypt(const unsigned char *plaintext, int plaintext_len, unsigned char *key,
unsigned char *iv, unsigned char *ciphertext) {
DES_key_schedule key_schedule;
DES_set_key_checked(key, &key_schedule);
DES_cbc_encrypt(plaintext, ciphertext, plaintext_len, &key_schedule, iv, DES_ENCRYPT);
}
// 解密函数
void decrypt(const unsigned char *ciphertext, int ciphertext_len, unsigned char *key,
unsigned char *iv, unsigned char *plaintext) {
DES_key_schedule key_schedule;
DES_set_key_checked(key, &key_schedule);
DES_cbc_encrypt(ciphertext, plaintext, ciphertext_len, &key_schedule, iv, DES_DECRYPT);
}
int main() {
// 明文
unsigned char plaintext[] = "Hello, world!";
int plaintext_len = strlen((char*)plaintext);
// 密钥
unsigned char key[] = "12345678";
unsigned char iv[] = "12345678";
// 分配空间
unsigned char ciphertext[1024];
unsigned char decryptedtext[1024];
// 加密
encrypt(plaintext, plaintext_len, key, iv, ciphertext);
// 输出密文
cout << "Ciphertext is: " << ciphertext << endl;
// 解密
decrypt(ciphertext, plaintext_len, key, iv, decryptedtext);
// 输出明文
cout << "Decrypted text is: " << decryptedtext << endl;
return 0;
}
```
在上面的代码中,我们使用了OpenSSL库中的DES函数进行加密和解密操作。在加密函数中,我们首先调用DES_set_key_checked函数设置密钥,然后调用DES_cbc_encrypt函数进行加密操作。在解密函数中,我们同样首先调用DES_set_key_checked函数设置密钥,然后调用DES_cbc_encrypt函数进行解密操作。
在main函数中,我们首先定义了明文、密钥和初始化向量,然后分配了密文和解密后的明文的空间。我们调用encrypt函数进行加密操作,然后输出密文。接着,我们调用decrypt函数进行解密操作,最后输出解密后的明文。
需要注意的是,在实际使用中,我们需要考虑密钥的安全性和保密性,以及加密模式、填充方式等问题,以确保信息加密的安全性和正确性。
阅读全文
相关推荐



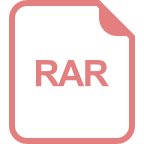

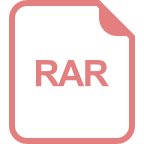

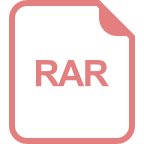





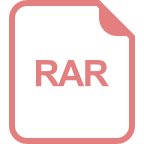




