set int array time[n + 1][n + 1] to INT_MAX, what function to achieve?
时间: 2024-02-29 15:51:40 浏览: 70
To set a 2D integer array `time[n+1][n+1]` to `INT_MAX`, you can use nested loops along with the `memset` function or the `fill_n` function.
Here's an example using `memset`:
```c++
#include <cstring>
#include <climits>
// ...
int n = 10; // example size
int time[n+1][n+1];
for (int i = 0; i <= n; i++) {
memset(time[i], INT_MAX, sizeof(time[i]));
}
```
And here's an example using `fill_n`:
```c++
#include <algorithm>
#include <climits>
// ...
int n = 10; // example size
int time[n+1][n+1];
for (int i = 0; i <= n; i++) {
std::fill_n(time[i], n+1, INT_MAX);
}
```
Both of these approaches accomplish the same thing: filling each element of `time` with the value `INT_MAX`. The first approach uses `memset` to set each row to `INT_MAX`, and the second approach uses `std::fill_n` to set each row to `INT_MAX`.
阅读全文
相关推荐
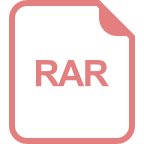
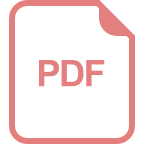
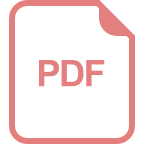
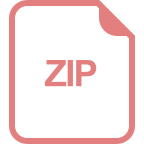
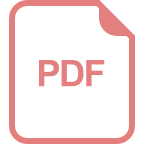
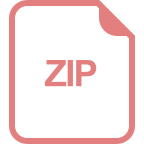
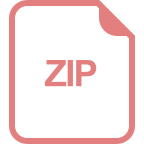
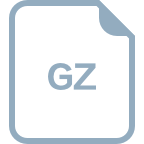
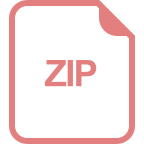
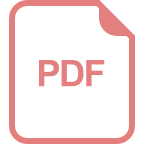
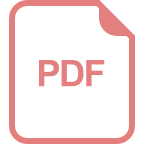
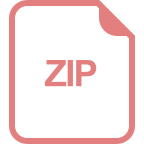
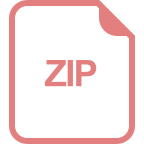
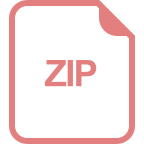
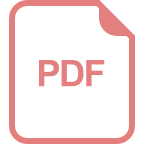
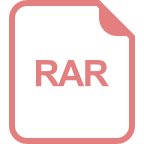
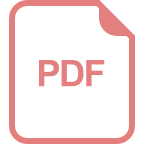