mfc 绘制一个线段的垂直相交线段,区分象限
时间: 2024-03-17 07:40:42 浏览: 67
要绘制一个线段的垂直相交线段,并区分在哪个象限,可以按照以下步骤进行:
1. 首先,获取原始线段的起点和终点坐标。
2. 然后,计算出原始线段的斜率,如果斜率不存在,则说明线段是垂直于 x 轴或 y 轴的。
3. 如果斜率不存在,可以通过起点坐标和终点坐标进行比较,确定垂直线段的起点和终点坐标,并判断在哪个象限。
4. 如果斜率存在,可以根据斜率和起点坐标计算出垂直线段的斜率。
5. 然后,根据斜率和起点坐标,以及垂直线段的长度,计算出垂直线段的终点坐标,并判断在哪个象限。
6. 最后,使用绘图函数,将原始线段和垂直线段绘制出来,并在垂直线段的起点处绘制一个小圆点,表示垂直线段所在的象限。
示例代码如下:
```cpp
void CMyView::DrawPerpendicularLineWithQuadrant(CDC* pDC, CPoint ptStart, CPoint ptEnd, int nLength)
{
// 计算原始线段的斜率
double dSlope = (double)(ptEnd.y - ptStart.y) / (double)(ptEnd.x - ptStart.x);
// 判断在哪个象限
int nQuadrant = 0;
if (ptStart.x < ptEnd.x && ptStart.y < ptEnd.y) // 第一象限
nQuadrant = 1;
else if (ptStart.x > ptEnd.x && ptStart.y < ptEnd.y) // 第二象限
nQuadrant = 2;
else if (ptStart.x > ptEnd.x && ptStart.y > ptEnd.y) // 第三象限
nQuadrant = 3;
else if (ptStart.x < ptEnd.x && ptStart.y > ptEnd.y) // 第四象限
nQuadrant = 4;
// 如果斜率不存在,说明线段垂直于 x 轴或 y 轴
if (dSlope == 0 || _isnan(dSlope))
{
if (ptStart.y == ptEnd.y) // 线段水平
{
// 计算垂直线段的起点和终点坐标
CPoint ptPerpStart(ptStart.x, ptStart.y - nLength / 2);
CPoint ptPerpEnd(ptEnd.x, ptEnd.y - nLength / 2);
// 绘制垂直线段
pDC->MoveTo(ptPerpStart);
pDC->LineTo(ptPerpEnd);
// 绘制象限标识
DrawQuadrant(pDC, ptPerpStart, nQuadrant);
}
else if (ptStart.x == ptEnd.x) // 线段垂直
{
// 计算垂直线段的起点和终点坐标
CPoint ptPerpStart(ptStart.x - nLength / 2, ptStart.y);
CPoint ptPerpEnd(ptEnd.x - nLength / 2, ptEnd.y);
// 绘制垂直线段
pDC->MoveTo(ptPerpStart);
pDC->LineTo(ptPerpEnd);
// 绘制象限标识
DrawQuadrant(pDC, ptPerpStart, nQuadrant);
}
}
else // 线段斜率存在
{
// 计算垂直线段的斜率
double dPerpSlope = -1 / dSlope;
// 计算垂直线段的长度
int nPerpLength = (int)sqrt(nLength * nLength / (1 + dPerpSlope * dPerpSlope));
// 计算垂直线段的起点和终点坐标
int nDeltaX = (int)(nPerpLength / sqrt(1 + dPerpSlope * dPerpSlope));
int nDeltaY = (int)(dPerpSlope * nDeltaX);
CPoint ptPerpStart(ptStart.x - nDeltaX, ptStart.y - nDeltaY);
CPoint ptPerpEnd(ptStart.x + nDeltaX, ptStart.y + nDeltaY);
// 绘制垂直线段
pDC->MoveTo(ptPerpStart);
pDC->LineTo(ptPerpEnd);
// 绘制象限标识
DrawQuadrant(pDC, ptPerpStart, nQuadrant);
}
}
void CMyView::DrawQuadrant(CDC* pDC, CPoint pt, int nQuadrant)
{
// 计算象限圆点的坐标
int nRadius = 5;
CPoint ptQuadrant(pt.x + nRadius, pt.y - nRadius);
switch (nQuadrant)
{
case 1:
ptQuadrant.Offset(nRadius, -nRadius);
break;
case 2:
ptQuadrant.Offset(-nRadius, -nRadius);
break;
case 3:
ptQuadrant.Offset(-nRadius, nRadius);
break;
case 4:
ptQuadrant.Offset(nRadius, nRadius);
break;
}
// 绘制象限圆点
pDC->Ellipse(ptQuadrant.x - nRadius, ptQuadrant.y - nRadius,
ptQuadrant.x + nRadius, ptQuadrant.y + nRadius);
}
```
这是一个 MFC 中的绘图函数,可以在视图类的 OnDraw 函数中调用。其中,pDC 是 CDC 对象,ptStart 和 ptEnd 是线段的起点和终点坐标,nLength 是垂直线段的长度。根据原始线段的斜率和坐标,计算出垂直线段的起点和终点坐标,并判断在哪个象限。然后使用绘图函数绘制出原始线段和垂直线段,并在垂直线段的起点处绘制一个小圆点,表示垂直线段所在的象限。
阅读全文
相关推荐









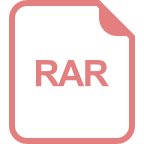


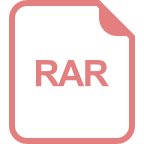




