已知一个dimension行len列的double型数组,请用C语言构建哈希表存储该数组的第dimension行,用户通过键盘输入数字在数组中查找该数字,如果查找不到则将输入的数字添加到原有哈希表中
时间: 2024-05-04 21:16:55 浏览: 81
以下是一个基本的哈希表实现,可以用来存储double型数组的第dimension行:
```c
#include <stdio.h>
#include <stdlib.h>
#define TABLE_SIZE 10000 //哈希表的大小
typedef struct ListNode {
double value;
struct ListNode* next;
} ListNode;
typedef struct HashTable {
ListNode* table[TABLE_SIZE];
int count;
} HashTable;
unsigned int hash(double value) {
return (unsigned int)value % TABLE_SIZE;
}
void insert(HashTable* ht, double value) {
unsigned int index = hash(value);
ListNode* node = ht->table[index];
while (node != NULL) {
if (node->value == value) {
return; //值已经存在,无需插入
}
node = node->next;
}
//值不存在,插入新节点
node = (ListNode*)malloc(sizeof(ListNode));
node->value = value;
node->next = ht->table[index];
ht->table[index] = node;
ht->count++;
}
ListNode* find(HashTable* ht, double value) {
unsigned int index = hash(value);
ListNode* node = ht->table[index];
while (node != NULL) {
if (node->value == value) {
return node; //找到了节点
}
node = node->next;
}
return NULL; //未找到节点
}
void initHashTable(HashTable* ht) {
for (int i = 0; i < TABLE_SIZE; i++) {
ht->table[i] = NULL;
}
ht->count = 0;
}
void freeHashTable(HashTable* ht) {
for (int i = 0; i < TABLE_SIZE; i++) {
ListNode* node = ht->table[i];
while (node != NULL) {
ListNode* temp = node;
node = node->next;
free(temp);
}
}
ht->count = 0;
}
int main() {
int dimension = 10;
int len = 20;
double arr[dimension][len];
//初始化数组
for (int i = 0; i < dimension; i++) {
for (int j = 0; j < len; j++) {
arr[i][j] = (double)rand();
}
}
//构建哈希表
HashTable ht;
initHashTable(&ht);
for (int j = 0; j < len; j++) {
insert(&ht, arr[dimension-1][j]); //将第dimension行的数据插入哈希表
}
//查询
double num;
printf("请输入要查找的数字:");
scanf("%lf", &num);
ListNode* node = find(&ht, num);
if (node == NULL) {
insert(&ht, num); //如果找不到则插入新节点
printf("已将该数字加入哈希表\n");
} else {
printf("找到了该数字\n");
}
//释放哈希表
freeHashTable(&ht);
return 0;
}
```
说明:
- 该哈希表使用链表法解决哈希冲突。
- hash函数使用取模运算将double型数字转换为unsigned int类型的哈希值。
- insert函数将新的double型数字插入哈希表中,如果该数字已经存在则不插入。
- find函数在哈希表中查找指定的double型数字,如果找到了则返回对应的节点,否则返回NULL。
- main函数中先将double型数组第dimension行的数据插入哈希表中,然后让用户输入数字进行查找,如果找不到则插入新节点。最后释放哈希表中的所有节点。
阅读全文
相关推荐
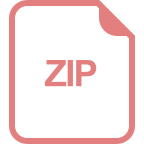
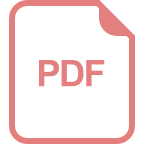
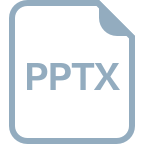
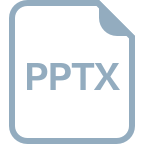
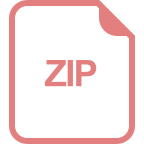
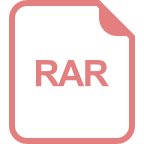
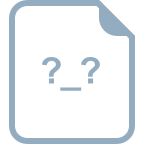
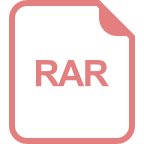
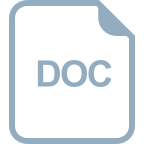
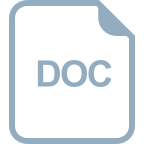





