创建一个cairo surface,这个surface是什么意思
时间: 2023-08-05 18:52:02 浏览: 254
Cairo是一个开源的2D图形库,可以用来创建各种类型的图形,如线条、矩形、圆形、多边形等等。而Cairo Surface则是Cairo库中的一个概念,它表示一个可绘制的目标,可以是内存中的图像、屏幕、PDF文档等,它提供了一个统一的接口来描述这些不同类型的目标,使得我们可以使用相同的API来绘制不同类型的图形。
因此,创建一个Cairo Surface就是在内存中或者其他目标上创建一个可以被Cairo库绘制的目标,这个目标可以用来绘制各种类型的图形。
相关问题
cairo 缩放 surface
要在 Cairo 中缩放一个 surface,可以使用 `cairo_scale()` 函数。该函数接受两个参数,分别是 X 和 Y 方向上的比例因子。例如,如果想将一个 surface 放大两倍,则可以使用以下代码:
```
cairo_scale(cr, 2.0, 2.0);
cairo_set_source_surface(cr, surface, 0, 0);
cairo_paint(cr);
```
其中,`cr` 是 Cairo 绘图上下文对象,`surface` 是要绘制的 surface 对象。在调用 `cairo_scale()` 函数之后,所有绘制操作都会按照指定的比例因子进行缩放。在这个例子中,`cairo_set_source_surface()` 函数将 surface 设置为当前的绘图源,然后使用 `cairo_paint()` 函数将其绘制出来。
cairo自定义surface
Cairo是一个功能强大的2D图形库,它支持多种绘图表面(surface)。其中,通过自定义surface,我们可以实现自己的绘图目的。
在Cairo中,Surface是一个表示要绘制的目标的抽象概念。Surface可以是一个窗口、一个图片文件、一个PDF文件等等。要创建一个自定义的surface对象,你需要定义一个Cairo_surface_t结构体,该结构体包含了一些函数指针,用于绑定你的自定义surface对象与Cairo库中的相关函数。
下面是一些示例代码,用于创建一个自定义的surface对象:
```
#include <cairo.h>
typedef struct _MySurface {
cairo_surface_t base;
// Define your own properties here
} MySurface;
static cairo_status_t my_surface_finish(cairo_surface_t *surface) {
// Define your own cleanup code here
return CAIRO_STATUS_SUCCESS;
}
static cairo_status_t my_surface_flush(cairo_surface_t *surface) {
// Define your own flush code here
return CAIRO_STATUS_SUCCESS;
}
static cairo_status_t my_surface_write_to_png(cairo_surface_t *surface, const char *filename) {
// Define your own code to write to PNG file here
return CAIRO_STATUS_SUCCESS;
}
static cairo_surface_t *my_surface_create_similar(cairo_surface_t *surface, cairo_content_t content, int width, int height) {
// Define your own code to create a similar surface here
return NULL;
}
static cairo_surface_t *my_surface_create_similar_image(cairo_surface_t *surface, cairo_format_t format, int width, int height) {
// Define your own code to create a similar image surface here
return NULL;
}
static cairo_surface_t *my_surface_create_for_rectangle(cairo_surface_t *surface, double x, double y, double width, double height) {
// Define your own code to create a surface for a rectangle here
return NULL;
}
static cairo_surface_t *my_surface_create_observer(cairo_surface_t *surface, cairo_surface_observer_mode_t mode) {
// Define your own code to create an observer surface here
return NULL;
}
static cairo_int_status_t my_surface_acquire_source_image(cairo_surface_t *surface, cairo_image_surface_t **image_out, void **extra_data_out) {
// Define your own code to acquire a source image here
return CAIRO_INT_STATUS_UNSUPPORTED;
}
static void my_surface_release_source_image(cairo_surface_t *surface, cairo_image_surface_t *image, void *extra_data) {
// Define your own code to release the source image here
}
static cairo_int_status_t my_surface_acquire_dest_image(cairo_surface_t *surface, cairo_rectangle_t *interest_rect, cairo_image_surface_t **image_out, cairo_rectangle_t *image_rect_out, void **extra_data_out) {
// Define your own code to acquire a destination image here
return CAIRO_INT_STATUS_UNSUPPORTED;
}
static void my_surface_release_dest_image(cairo_surface_t *surface, cairo_rectangle_t *interest_rect, cairo_image_surface_t *image, cairo_rectangle_t *image_rect, void *extra_data) {
// Define your own code to release the destination image here
}
static cairo_bool_t my_surface_get_extents(cairo_surface_t *surface, cairo_rectangle_t *rectangle) {
// Define your own code to get the extents of the surface here
return FALSE;
}
static cairo_surface_t *my_surface_map_to_image(cairo_surface_t *surface, cairo_rectangle_t *extents) {
// Define your own code to map the surface to an image here
return NULL;
}
static void my_surface_unmap_image(cairo_surface_t *surface, cairo_surface_t *image) {
// Define your own code to unmap the image here
}
static cairo_surface_t *my_surface_map_to_image_surface(cairo_surface_t *surface, cairo_rectangle_t *extents, cairo_format_t format, void **data_out, int *stride_out) {
// Define your own code to map the surface to an image surface here
return NULL;
}
static void my_surface_unmap_image_surface(cairo_surface_t *surface, cairo_surface_t *image_surface, void *data) {
// Define your own code to unmap the image surface here
}
static cairo_status_t my_surface_snapshot(cairo_surface_t *surface, cairo_surface_t **snapshot_out) {
// Define your own code to snapshot the surface here
return CAIRO_STATUS_SUCCESS;
}
static cairo_surface_t *my_surface_copy_page(cairo_surface_t *surface) {
// Define your own code to copy a page of the surface here
return NULL;
}
static cairo_status_t my_surface_show_page(cairo_surface_t *surface) {
// Define your own code to show a page of the surface here
return CAIRO_STATUS_SUCCESS;
}
static cairo_bool_t my_surface_has_show_text_glyphs(cairo_surface_t *surface) {
// Define your own code to determine if the surface supports show_text_glyphs here
return FALSE;
}
static cairo_status_t my_surface_show_text_glyphs(cairo_surface_t *surface, const char *utf8, int utf8_len, const cairo_glyph_t *glyphs, int num_glyphs, const cairo_text_cluster_t *clusters, int num_clusters, const cairo_text_cluster_flags_t *cluster_flags) {
// Define your own code to support show_text_glyphs here
return CAIRO_STATUS_SUCCESS;
}
static cairo_bool_t my_surface_supports_mime_type(cairo_surface_t *surface, const char *mime_type) {
// Define your own code to determine if the surface supports a MIME type here
return FALSE;
}
static cairo_status_t my_surface_set_mime_data(cairo_surface_t *surface, const char *mime_type, const unsigned char *data, unsigned long length, cairo_destroy_func_t destroy, void *closure) {
// Define your own code to set MIME data here
return CAIRO_STATUS_SUCCESS;
}
static cairo_status_t my_surface_get_font_options(cairo_surface_t *surface, cairo_font_options_t *options) {
// Define your own code to get font options here
return CAIRO_STATUS_SUCCESS;
}
static cairo_status_t my_surface_flush_image(cairo_surface_t *surface, cairo_surface_t *image_surface, cairo_rectangle_t *extents) {
// Define your own code to flush an image here
return CAIRO_STATUS_SUCCESS;
}
static const cairo_surface_backend_t my_surface_backend = {
CAIRO_SURFACE_TYPE_CUSTOM,
my_surface_finish,
my_surface_create_similar,
my_surface_map_to_image,
my_surface_unmap_image,
my_surface_acquire_source_image,
my_surface_release_source_image,
my_surface_acquire_dest_image,
my_surface_release_dest_image,
my_surface_clone_similar,
my_surface_composite,
my_surface_fill_rectangles,
my_surface_fill_region,
my_surface_set_device_offset,
my_surface_get_device_offset,
my_surface_set_fallback_resolution,
my_surface_get_fallback_resolution,
my_surface_copy_page,
my_surface_show_page,
my_surface_get_extents,
my_surface_has_show_text_glyphs,
my_surface_show_text_glyphs,
my_surface_set_mime_data,
my_surface_supports_mime_type,
my_surface_snapshot,
my_surface_copy_page,
my_surface_show_page,
my_surface_get_font_options,
my_surface_flush,
my_surface_flush_image,
my_surface_create_observer,
my_surface_create_similar_image,
my_surface_map_to_image_surface,
my_surface_unmap_image_surface
};
cairo_surface_t *my_surface_create() {
MySurface *surface = malloc(sizeof(MySurface));
surface->base.backend = &my_surface_backend;
// Initialize your own properties here
return &surface->base;
}
```
在上面的代码中,我们定义了一个MySurface结构体,它是cairo_surface_t的子类。我们还定义了一些函数,这些函数将用于绑定我们的自定义surface对象与Cairo库中的相关函数。
最后,我们定义了一个my_surface_create函数,该函数将创建并返回我们的自定义surface对象。
要使用自定义surface,只需将其传递给cairo_create函数即可:
```
cairo_surface_t *surface = my_surface_create();
cairo_t *cr = cairo_create(surface);
```
现在,我们已经成功地创建了一个自定义的Cairo绘图表面(surface),并且可以使用它来进行绘制操作。
阅读全文
相关推荐
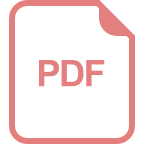
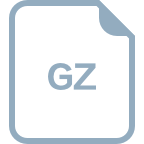
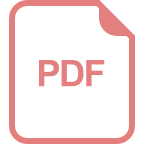
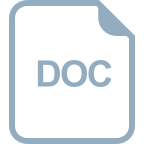
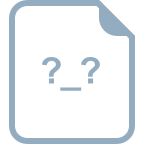
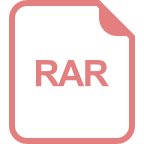
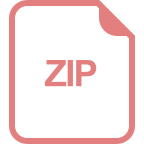
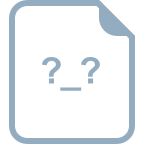
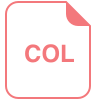
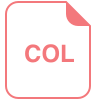





