itextsharp word转pdf
时间: 2023-08-31 19:05:15 浏览: 72
iTextSharp 是一个 C# 的 PDF 生成工具库,可以使用它将 Word 文档转换为 PDF 文件。下面是使用 iTextSharp 实现 Word 转 PDF 的示例代码:
```csharp
using System;
using System.IO;
using iTextSharp.text;
using iTextSharp.text.pdf;
using Microsoft.Office.Interop.Word;
namespace WordToPdf
{
class Program
{
static void Main(string[] args)
{
string inputFilePath = @"C:\input.docx";
string outputFilePath = @"C:\output.pdf";
// 创建 Word 应用程序对象
Application word = new Application();
// 打开 Word 文档
object inputFile = inputFilePath;
object nullObject = System.Reflection.Missing.Value;
Document doc = word.Documents.Open(ref inputFile, ref nullObject, ref nullObject,
ref nullObject, ref nullObject, ref nullObject, ref nullObject, ref nullObject,
ref nullObject, ref nullObject, ref nullObject, ref nullObject, ref nullObject,
ref nullObject, ref nullObject, ref nullObject);
// 创建 PDF 文档对象
Document pdfDoc = new Document();
// 创建 PDF 编写器
PdfWriter.GetInstance(pdfDoc, new FileStream(outputFilePath, FileMode.Create));
// 打开 PDF 文档
pdfDoc.Open();
// 将 Word 文档内容写入 PDF 文档
foreach (Section section in doc.Sections)
{
foreach (Microsoft.Office.Interop.Word.Paragraph paragraph in section.Paragraphs)
{
foreach (Microsoft.Office.Interop.Word.Range range in paragraph.Range.StoryRanges)
{
string text = range.Text;
pdfDoc.Add(new Paragraph(text));
}
}
}
// 关闭 PDF 文档
pdfDoc.Close();
// 关闭 Word 文档和应用程序
doc.Close(ref nullObject, ref nullObject, ref nullObject);
word.Quit(ref nullObject, ref nullObject, ref nullObject);
}
}
}
```
在代码中,使用 `Application` 类创建了一个 Word 应用程序对象,并使用 `Documents.Open` 方法打开了要转换的 Word 文档。然后创建了一个 iTextSharp 的 `Document` 对象,并使用 `PdfWriter` 创建了一个 PDF 编写器。接着使用 `pdfDoc.Add` 方法将 Word 文档内容写入 PDF 文档中。最后关闭了 PDF 文档、Word 文档和应用程序。
需要注意的是,上述示例代码需要引用 iTextSharp 和 Microsoft.Office.Interop.Word 两个库。此外,如果没有安装 Office,可能需要安装 Office 互操作性组件(Microsoft Office Interop assemblies)。
阅读全文
相关推荐

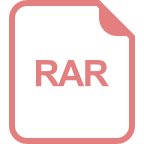



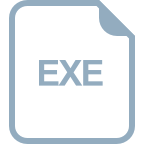
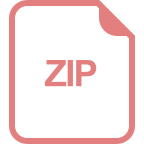
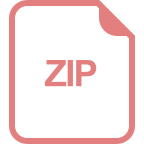