mounted() { let that = this; that.$websocket.registerEvent("event.sensor", (data) => { let resultMessage = JSON.parse(data); this.sensorInfoList = resultMessage }); },解释以上代码
时间: 2024-04-25 08:26:16 浏览: 20
这段代码是一个Vue组件生命周期函数`mounted()`,当组件挂载到DOM上时,将会执行这个函数。函数内部定义了一个变量`that`,它指向组件对象`this`,这么做是为了在后面的回调函数中访问组件的数据。接着,使用`$websocket`插件的`registerEvent()`方法注册了一个名为`event.sensor`的事件,当有这个事件发生时,会执行回调函数,回调函数内部将收到的数据`data`转换为JSON格式的对象,并将它赋值给组件的`sensorInfoList`数据。这段代码的作用是将WebSocket接收到的`event.sensor`事件数据更新到组件的`sensorInfoList`数据中。
相关问题
let video = this.$refs.videoElement; video为空如何处理
如果 `this.$refs.videoElement` 返回为空,有几种可能的原因和解决方法:
1. 确保在模板中正确地设置了 `ref` 属性,并且与 `this.$refs` 中的引用名称一致。
```html
<template>
<div>
<video ref="videoElement"></video>
</div>
</template>
```
2. 确保在访问 `this.$refs.videoElement` 之前,已经渲染了包含该元素的组件。Vue 组件的生命周期钩子函数 `mounted` 是一个常用的地方,可以在其中访问 `$refs`。
```javascript
<script>
export default {
mounted() {
const video = this.$refs.videoElement;
if (video) {
// 在这里可以操作 video 元素
} else {
console.log("videoElement is not available");
}
}
}
</script>
```
3. 如果你是在条件渲染(`v-if`)的情况下使用 `ref`,则需要确保条件满足时才能访问 `$refs`。
```html
<template>
<div>
<div v-if="showVideo">
<video ref="videoElement"></video>
</div>
<button @click="showVideo = true">Show Video</button>
</div>
</template>
<script>
export default {
data() {
return {
showVideo: false
};
},
mounted() {
if (this.showVideo) {
const video = this.$refs.videoElement;
if (video) {
// 在这里可以操作 video 元素
} else {
console.log("videoElement is not available");
}
}
}
}
</script>
```
通过以上的处理,你应该能够正确地获取到 `this.$refs.videoElement`,并且进行后续的操作。
this.$nextTick(() => { this.$refs.weightInput.select(); });页面报错_this3.$refs.weightInput.select is not a function"
如果页面报错 `this.$refs.weightInput.select is not a function`,可能是因为 `$refs` 中的元素是一个组件,而不是原生的 DOM 元素。
如果您使用的是第三方组件库,建议您查看该组件库的文档,了解如何正确地获取组件中的输入框元素并执行选中文本的操作。
如果您自己编写了组件,可以在组件的 `mounted` 钩子函数中获取输入框元素并保存到实例中,然后在需要选中文本的时候调用该元素的 `select` 方法。
具体实现步骤如下:
1. 在组件中定义一个 `inputRef` 属性,用于保存输入框元素的引用。
```javascript
export default {
data() {
return {
weight: '',
inputRef: null,
};
},
mounted() {
this.inputRef = this.$refs.weightInput.$el.querySelector('input');
},
methods: {
selectText() {
this.$nextTick(() => {
this.inputRef.select();
});
},
},
};
```
2. 在组件的模板中给输入框组件添加 `ref` 属性,并在 `mounted` 钩子函数中获取输入框元素的引用。
```html
<template>
<div>
<my-input ref="weightInput" v-model="weight"></my-input>
</div>
</template>
```
通过在组件的 `mounted` 钩子函数中获取输入框元素,并在需要选中文本的时候调用该元素的 `select` 方法,就可以实现选中文本的功能。
希望对您有所帮助!
相关推荐
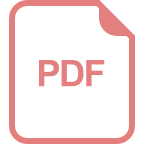
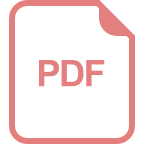
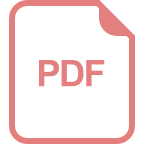












