C#一个图标随机出现在几个不同的位置,随机移动,点击图标则显示图标的另一种形态,并加上音效和背景音乐
时间: 2024-03-16 19:45:06 浏览: 33
这个问题需要分几个步骤来实现,具体步骤如下:
1. 随机图标出现的位置
使用 C# 的 Random 类生成随机数来确定图标出现的位置,可以使用 System.Windows.Forms.Control 类的 Location 属性来设置图标的位置,示例代码如下:
```csharp
Random rand = new Random();
int x = rand.Next(this.ClientSize.Width - icon.Width);
int y = rand.Next(this.ClientSize.Height - icon.Height);
icon.Location = new Point(x, y);
```
其中 `this.ClientSize` 表示当前窗口的客户区大小,`icon` 是一个 PictureBox 控件,表示图标。
2. 随机移动图标
使用 Timer 控件来定时移动图标,可以在 Tick 事件中随机改变图标的位置,示例代码如下:
```csharp
private void timer_Tick(object sender, EventArgs e)
{
Random rand = new Random();
int x = rand.Next(this.ClientSize.Width - icon.Width);
int y = rand.Next(this.ClientSize.Height - icon.Height);
icon.Location = new Point(x, y);
}
```
3. 点击图标显示另一种形态
使用 PictureBox 控件的 Click 事件来响应图标的点击事件,可以在事件处理程序中改变图标的 Image 属性,示例代码如下:
```csharp
private void icon_Click(object sender, EventArgs e)
{
icon.Image = Properties.Resources.another_icon;
}
```
其中 `Properties.Resources.another_icon` 表示另一种图标的资源文件。
4. 加上音效和背景音乐
使用 System.Media.SoundPlayer 类来播放音效和背景音乐,可以在相应的事件处理程序中调用 Play 方法来播放音乐,示例代码如下:
```csharp
SoundPlayer player = new SoundPlayer(Properties.Resources.sound_effect);
player.Play();
```
其中 `Properties.Resources.sound_effect` 表示音效的资源文件。
完整的代码如下:
```csharp
public partial class Form1 : Form
{
private PictureBox icon;
private Timer timer;
public Form1()
{
InitializeComponent();
icon = new PictureBox();
icon.Image = Properties.Resources.icon;
icon.Size = icon.Image.Size;
icon.Location = new Point(0, 0);
icon.Click += icon_Click;
this.Controls.Add(icon);
timer = new Timer();
timer.Interval = 1000;
timer.Tick += timer_Tick;
timer.Start();
}
private void icon_Click(object sender, EventArgs e)
{
icon.Image = Properties.Resources.another_icon;
SoundPlayer player = new SoundPlayer(Properties.Resources.sound_effect);
player.Play();
}
private void timer_Tick(object sender, EventArgs e)
{
Random rand = new Random();
int x = rand.Next(this.ClientSize.Width - icon.Width);
int y = rand.Next(this.ClientSize.Height - icon.Height);
icon.Location = new Point(x, y);
SoundPlayer player = new SoundPlayer(Properties.Resources.background_music);
player.PlayLooping();
}
}
```
其中 `Properties.Resources.background_music` 表示背景音乐的资源文件。
相关推荐
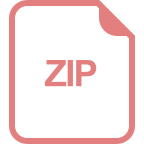
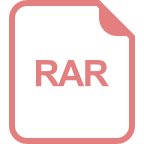














