”public static long[] getTopUsers(int howMany, LongPrimitiveIterator allUserIDs, Estimator<Long> estimator) throws Exception { Queue<SimilarUser> topUsers = new PriorityQueue<>(howMany + 1, Collections.reverseOrder()); boolean full = false; double lowestTopValue = Double.NEGATIVE_INFINITY; while (allUserIDs.hasNext()) { long userID = allUserIDs.next(); double similarity; try { similarity = estimator.estimate(userID); } catch (Exception nsue) { continue; } if (!Double.isNaN(similarity) && (!full || similarity > lowestTopValue)) { topUsers.add(new SimilarUser(userID, similarity)); if (full) { topUsers.poll(); } else if (topUsers.size() > howMany) { full = true; topUsers.poll(); } SimilarUser similarUser = topUsers.peek(); if (similarUser != null) { lowestTopValue = topUsers.peek().getSimilarity(); } } } int size = topUsers.size(); if (size == 0) { return NO_IDS; } List<SimilarUser> sorted = new ArrayList<>(size); sorted.addAll(topUsers); Collections.sort(sorted); long[] result = new long[size]; int i = 0; for (SimilarUser similarUser : sorted) { result[i++] = similarUser.getUserID(); } return result; }“ 解释嗲吗
时间: 2023-12-03 18:02:39 浏览: 74
这段代码是实现了一个获取相似用户的方法,输入参数包括需要获取的相似用户数量howMany、所有用户ID的迭代器allUserIDs、以及一个用于估计用户相似度的Estimator接口实现类estimator。方法通过遍历所有用户ID,对每个用户使用estimator估计出与目标用户的相似度,并将相似度和用户ID以SimilarUser对象的形式加入到一个优先队列topUsers中。当topUsers的大小超过howMany时,会将相似度最小的用户从队列中移除。最终将topUsers转换为按相似度从大到小排序的List,并将用户ID存入long型数组result中返回。如果无法估计某个用户的相似度,会跳过该用户,如果没有找到任何相似用户,会返回一个空数组NO_IDS。
相关问题
“ public static List<RecommendedItem> getTopItems(int howMany, LongPrimitiveIterator possibleItemIDs, Estimator<Long> estimator) throws Exception { Preconditions.checkArgument(possibleItemIDs != null, "possibleItemIDs is null"); Preconditions.checkArgument(estimator != null, "estimator is null"); Queue<RecommendedItem> topItems = new PriorityQueue<>(howMany + 1,Collections.reverseOrder()); boolean full = false; double lowestTopValue = Double.NEGATIVE_INFINITY; while (possibleItemIDs.hasNext()) { long itemID = possibleItemIDs.next(); double preference; try { preference = estimator.estimate(itemID); } catch (Exception nsie) { continue; } if (!Double.isNaN(preference) && (!full || preference > lowestTopValue)) { topItems.add(new GenericRecommendedItem(itemID, (float) preference)); if (full) { topItems.poll(); } else if (topItems.size() > howMany) { full = true; topItems.poll(); } RecommendedItem similarUser = topItems.peek(); if (similarUser != null) { lowestTopValue = topItems.peek().getValue(); } } } int size = topItems.size(); if (size == 0) { return Collections.emptyList(); } List<RecommendedItem> result = new ArrayList<>(size); result.addAll(topItems); Collections.sort(result); return result; }” 解释代码
这段代码是一个用于获取推荐物品列表的方法。它接受三个参数:推荐物品的数量howMany、物品ID的迭代器possibleItemIDs和评估器estimator。其中,评估器estimator是用于计算物品偏好值的,它需要实现Estimator接口。
该方法使用优先队列来维护当前已发现的最好的推荐物品列表,通过遍历possibleItemIDs中的物品ID,并使用estimator计算物品的偏好值。如果该物品的偏好值大于当前最低评分(即topItems队列中最小的评分),那么就将该物品加入到topItems队列中,并更新最低评分。如果topItems队列已满,则将最差的物品从队列中移除。
最后,将topItems队列中的物品按照评分从高到低排序,将其转换为RecommendedItem对象列表并返回。如果topItems队列为空,则返回一个空的列表。
"@Override public List<RecommendedItem> recommend(long userID, int howMany, boolean includeKnownItems) throws Exception { Preconditions.checkArgument(howMany >= 1, "howMany must be at least 1"); log.info("Recommending items for user ID '{}'", userID); // 获取邻居列表 long[] theNeighborhood = neighborhood.getUserNeighborhood(userID); if (theNeighborhood.length == 0) { return Collections.emptyList(); } FastIDSet allItemIDs = getAllOtherItems(theNeighborhood, userID, includeKnownItems); Estimator estimator = new Estimator(userID, theNeighborhood); List<RecommendedItem> topItems = TopItems.getTopItems(howMany, allItemIDs.iterator(), estimator); log.info("Recommendations are: {}", topItems); return topItems; }" 解释代码
这段代码是一个基于协同过滤的推荐算法中的推荐函数,它会为给定用户推荐一些物品。具体来说,这个函数会做以下几件事情:
1. 首先,它会检查输入的参数是否合法,确保需要返回的推荐物品数量 howMany 至少为 1。
2. 然后,它会打印一条日志,说明正在为用户 ID 为 userID 的用户进行推荐。
3. 接着,它会调用 neighborhood.getUserNeighborhood(userID) 函数,获取与该用户最相似的邻居列表 theNeighborhood。注意,这里的相似度是根据用户之间对物品的评分计算得出的。
4. 如果 theNeighborhood 为空,说明该用户没有任何邻居,直接返回一个空的推荐物品列表。
5. 否则,它会调用 getAllOtherItems 函数,获取所有邻居评分过的物品的 ID,存放在 allItemIDs 变量中。这里需要注意的是,如果 includeKnownItems 为 true,那么也会包括该用户评分过的物品。
6. 接下来,它会创建一个 Estimator 对象 estimator,用于预测该用户对某个物品的评分。
7. 然后,它会调用 TopItems.getTopItems 函数,获取预测评分最高的前 howMany 个物品,并将它们存放在 topItems 变量中。
8. 最后,它会再次打印一条日志,说明推荐的物品列表是什么,并返回 topItems。
总的来说,这段代码实现了一个简单的基于用户的协同过滤推荐算法,它假设用户之间的评分具有相似性,根据相似的邻居的评分,预测该用户对某个物品的评分,并推荐评分最高的几个物品。
阅读全文
相关推荐
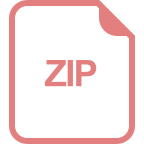
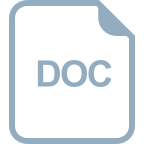
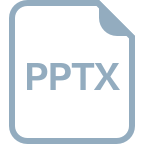
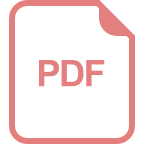
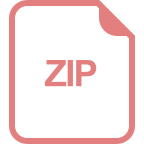
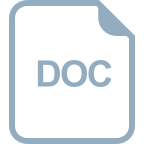
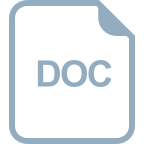
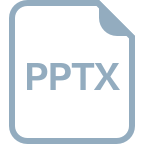
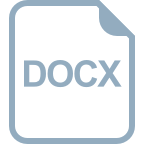
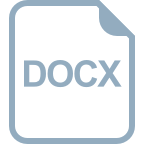
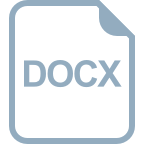
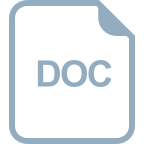

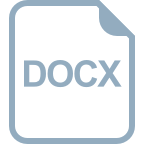