bytetrack算法结合光流法做跟踪的python代码
时间: 2023-12-22 08:03:27 浏览: 31
ByteTrack是一种基于目标检测的目标跟踪算法,结合光流法可以进一步提高跟踪的准确性和鲁棒性。下面是一个简单的Python实现示例:
```python
import numpy as np
import cv2
# 加载模型和配置文件
model = cv2.dnn_DetectionModel('models/ByteTrack.onnx')
model.setInputParams(size=(640, 640), scale=1/255, swapRB=True)
# 初始化光流法参数
lk_params = dict(winSize=(15, 15), maxLevel=2, criteria=(cv2.TERM_CRITERIA_EPS | cv2.TERM_CRITERIA_COUNT, 10, 0.03))
# 读取视频
cap = cv2.VideoCapture('video.mp4')
# 读取第一帧图像
ret, frame = cap.read()
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
# 检测目标ROI区域
results = model.detect(gray, confThreshold=0.5)
if len(results) > 0:
bbox = results[0][2]
bbox = (bbox[0], bbox[1], bbox[2] - bbox[0], bbox[3] - bbox[1])
r, h, c, w = bbox
else:
raise ValueError('No object detected in the first frame!')
# 初始化光流法追踪点
old_points = np.array([[c + w / 2, r + h / 2]], dtype=np.float32)
while True:
# 读取当前帧图像
ret, frame = cap.read()
if not ret:
break
gray_next = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
# 计算光流向量
new_points, status, error = cv2.calcOpticalFlowPyrLK(gray, gray_next, old_points, None, **lk_params)
# 筛选出成功跟踪的点
good_new = new_points[status == 1]
good_old = old_points[status == 1]
# 计算目标的位置和速度
if len(good_new) > 0:
bbox = (int(good_new[0][0] - w / 2), int(r), w, h)
results = model.detect(frame, bbox, confThreshold=0.5)
if len(results) > 0:
bbox = results[0][2]
bbox = (bbox[0], bbox[1], bbox[2] - bbox[0], bbox[3] - bbox[1])
r, h, c, w = bbox
cv2.rectangle(frame, bbox, (0, 255, 0), 2)
else:
cv2.putText(frame, 'Lost', (c, r - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.9, (0, 0, 255), 2)
# 更新光流法追踪点
old_points = good_new.reshape(-1, 1, 2)
# 显示结果
cv2.imshow("Frame", frame)
if cv2.waitKey(1) == 27:
break
# 释放资源
cap.release()
cv2.destroyAllWindows()
```
注意:这只是一个简单的示例,实际应用中,ByteTrack算法和光流法的参数需要进行更多的优化和改进,以提高目标跟踪的准确性和鲁棒性。
相关推荐
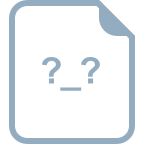














