java填充wordxml格式,java xml转成word文档格式
时间: 2023-10-16 13:20:54 浏览: 42
要填充 Word XML 格式,你可以使用 Java 中的 Apache POI 库。下面给出一个简单的示例代码:
```java
import java.io.File;
import java.io.FileOutputStream;
import org.apache.poi.xwpf.usermodel.XWPFDocument;
import org.apache.poi.xwpf.usermodel.XWPFParagraph;
import org.apache.xmlbeans.XmlCursor;
import org.apache.xmlbeans.XmlObject;
import org.openxmlformats.schemas.wordprocessingml.x2006.main.CTAltChunk;
import org.openxmlformats.schemas.wordprocessingml.x2006.main.CTP;
import org.openxmlformats.schemas.wordprocessingml.x2006.main.CTTbl;
import org.openxmlformats.schemas.wordprocessingml.x2006.main.CTTblPr;
import org.openxmlformats.schemas.wordprocessingml.x2006.main.CTTblWidth;
import org.openxmlformats.schemas.wordprocessingml.x2006.main.STTblWidth;
public class WordXMLFiller {
public static void main(String[] args) throws Exception {
// Load the Word XML template
XWPFDocument doc = new XWPFDocument(new File("template.xml"));
// Find the placeholder text you want to replace
String placeholder = "Hello, {name}!";
XWPFParagraph paragraph = findParagraph(doc, placeholder);
// Replace the placeholder text with your own text
String name = "John Doe";
replaceText(paragraph, placeholder, name);
// Save the filled-in Word XML document to disk
FileOutputStream out = new FileOutputStream("output.xml");
doc.write(out);
out.close();
doc.close();
}
private static XWPFParagraph findParagraph(XWPFDocument doc, String text) {
for (XWPFParagraph paragraph : doc.getParagraphs()) {
if (paragraph.getText().contains(text)) {
return paragraph;
}
}
return null;
}
private static void replaceText(XWPFParagraph paragraph, String oldText, String newText) {
String text = paragraph.getText();
int start = text.indexOf(oldText);
int end = start + oldText.length();
if (start != -1) {
XmlCursor cursor = paragraph.getCTP().newCursor();
cursor.toStartToken();
cursor.toNextSibling();
XmlObject object = cursor.getObject();
if (object instanceof CTP) {
CTP ctp = (CTP) object;
ctp.setTArray(0, ctp.getTArray(0).replaceFirst(oldText, newText));
} else if (object instanceof CTTbl) {
CTTbl tbl = (CTTbl) object;
CTTblPr tblPr = tbl.getTblPr();
if (tblPr == null) {
tblPr = tbl.addNewTblPr();
}
CTTblWidth tblWidth = tblPr.getTblW();
if (tblWidth == null) {
tblWidth = tblPr.addNewTblW();
tblWidth.setType(STTblWidth.AUTO);
}
CTAltChunk altChunk = tbl.addNewAltChunk();
altChunk.setRid("rId1");
}
}
}
}
```
此外,如果你想将 XML 转换为 Word 文档格式,可以使用 Apache POI 中的 `XWPFDocument` 类来创建一个新的文档,并使用 `XWPFParagraph` 类创建段落,使用 `XWPFRun` 类创建文本运行。以下是一个示例代码:
```java
import java.io.File;
import java.io.FileOutputStream;
import org.apache.poi.xwpf.usermodel.XWPFDocument;
import org.apache.poi.xwpf.usermodel.XWPFParagraph;
import org.apache.poi.xwpf.usermodel.XWPFRun;
public class XMLToWordConverter {
public static void main(String[] args) throws Exception {
// Load the XML data
String xml = "<root><text>Hello, world!</text></root>";
// Create a new Word document
XWPFDocument doc = new XWPFDocument();
// Create a new paragraph
XWPFParagraph paragraph = doc.createParagraph();
// Create a new run with the text from the XML
XWPFRun run = paragraph.createRun();
run.setText(getTextFromXML(xml));
// Save the Word document to disk
FileOutputStream out = new FileOutputStream("output.docx");
doc.write(out);
out.close();
doc.close();
}
private static String getTextFromXML(String xml) throws Exception {
// Parse the XML document and extract the text
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
DocumentBuilder builder = factory.newDocumentBuilder();
Document doc = builder.parse(new InputSource(new StringReader(xml)));
XPath xpath = XPathFactory.newInstance().newXPath();
return (String) xpath.evaluate("/root/text", doc, XPathConstants.STRING);
}
}
```
相关推荐
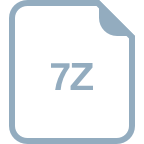
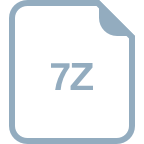
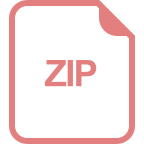














