用Java写,用短信猫替换腾讯云的代码
时间: 2024-04-21 13:26:57 浏览: 126
以下是一个使用Java短信猫替换腾讯云短信发送的示例代码:
```java
import java.util.ArrayList;
import java.util.List;
public class SMS {
// 短信猫串口号
private static final String PORT = "COM1";
// 短信猫接口
private SMSInterface smsInterface;
// 腾讯云短信API参数
private static final String SECRET_ID = "your_secret_id";
private static final String SECRET_KEY = "your_secret_key";
private static final String APP_ID = "your_app_id";
private static final String TEMPLATE_ID = "your_template_id";
public SMS() {
smsInterface = new SMSInterface();
smsInterface.initialize(PORT);
}
public void send(String phoneNumber, String message) {
// 短信猫发送
smsInterface.send(phoneNumber, message);
System.out.println("SMS sent via SMS interface.");
// 腾讯云短信发送,省略了签名和短信模板参数
try {
Credential cred = new Credential(SECRET_ID, SECRET_KEY);
SmsClient client = new SmsClient(cred, "ap-guangzhou");
SendSmsRequest req = new SendSmsRequest();
String[] phoneNumbers = { "+86" + phoneNumber };
req.setPhoneNumberSet(phoneNumbers);
req.setTemplateID(TEMPLATE_ID);
req.setTemplateParamSet(new String[] { message });
SendSmsResponse resp = client.SendSms(req);
System.out.println(resp.toJsonString());
} catch (TencentCloudSDKException e) {
System.out.println(e.toString());
}
}
public void close() {
smsInterface.close();
}
public static void main(String[] args) {
SMS sms = new SMS();
sms.send("12345678901", "Hello, world!");
sms.close();
}
}
```
这个示例代码首先初始化了一个短信猫接口,并使用它发送了一条短信。然后它使用腾讯云的Java SDK发送了一条短信,其中包括了API参数和短信内容。你需要将 `your_secret_id`、`your_secret_key`、`your_app_id`、`your_template_id` 替换为你自己的腾讯云API参数。最后,它关闭了短信猫接口。
阅读全文
相关推荐
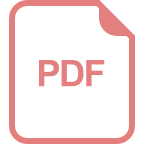
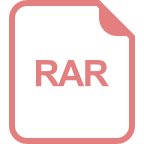
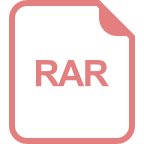
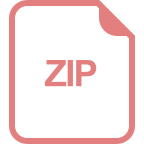


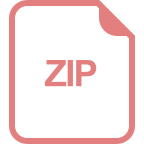
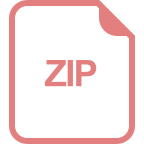
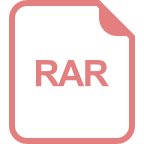
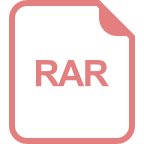

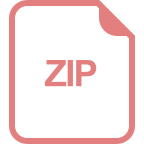
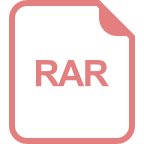
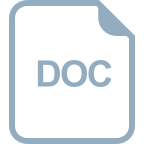

