用Java写一个微信扫码登录的接口
时间: 2023-05-24 17:04:38 浏览: 240
以下是使用Java实现微信扫码登录的示例代码:
首先,需要引入相关的依赖包:
```xml
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-core</artifactId>
<version>2.8.8</version>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.8.8</version>
</dependency>
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.5</version>
</dependency>
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpcore</artifactId>
<version>4.4.9</version>
</dependency>
```
然后,编写调用微信接口获取二维码的函数:
```java
public static String getQRCodeUrl() throws IOException {
CloseableHttpClient httpclient = HttpClients.createDefault();
HttpPost httpPost = new HttpPost(String.format("https://open.weixin.qq.com/connect/qrconnect?appid=%s&redirect_uri=%s&response_type=code&scope=snsapi_login#wechat_redirect",
APP_ID, REDIRECT_URI));
CloseableHttpResponse response = httpclient.execute(httpPost);
String html = EntityUtils.toString(response.getEntity());
Document doc = Jsoup.parse(html);
String qrUrl = doc.select("img[src^=\"//login.weixin.qq.com/qrcode/\"]").attr("src");
return "https:" + qrUrl;
}
```
接下来,在用户扫描二维码后,可以通过以下函数获取用户信息:
```java
public static String getUserInfo(String code) throws IOException {
CloseableHttpClient httpclient = HttpClients.createDefault();
HttpPost httpPost = new HttpPost(String.format("https://api.weixin.qq.com/sns/oauth2/access_token?appid=%s&secret=%s&code=%s&grant_type=authorization_code", APP_ID, APP_SECRET, code));
CloseableHttpResponse response = httpclient.execute(httpPost);
String jsonString = EntityUtils.toString(response.getEntity());
ObjectMapper objectMapper = new ObjectMapper();
JsonNode jsonNode = objectMapper.readTree(jsonString);
String accessToken = jsonNode.get("access_token").asText();
String openId = jsonNode.get("openid").asText();
HttpGet httpGet = new HttpGet(String.format("https://api.weixin.qq.com/sns/userinfo?access_token=%s&openid=%s&lang=zh_CN", accessToken, openId));
response = httpclient.execute(httpGet);
jsonString = EntityUtils.toString(response.getEntity());
return jsonString;
}
```
这个函数将返回JSON格式的用户信息。根据需要,我们还可以进一步解析JSON,获取用户昵称、头像等信息。
以上就是一个简单的微信扫码登录接口的实现示例。
相关推荐
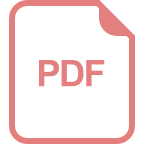
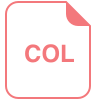
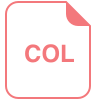
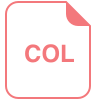
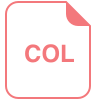
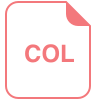









