c++输入一串文本,统计其中出现的词频(词的个数),并按字典序输出。 输入示例: I am a teacher 输出://输出格式:词和数字间空一格,数字后无空格 I 1 am 1 a 1 teacher 1
时间: 2023-06-14 09:05:52 浏览: 52
以下是一个简单的实现:
```cpp
#include <iostream>
#include <string>
#include <map>
#include <algorithm>
#include <cctype>
using namespace std;
int main() {
string text;
getline(cin, text);
map<string, int> freq_map;
// 将文本转换为小写字母形式,方便统计词频
transform(text.begin(), text.end(), text.begin(), ::tolower);
// 分割文本并统计词频
string word;
for (int i = 0; i < text.size(); i++) {
if (isalpha(text[i])) {
word += text[i];
} else {
if (word != "") {
freq_map[word]++;
word = "";
}
}
}
if (word != "") {
freq_map[word]++;
}
// 按字典序输出
for (auto it = freq_map.begin(); it != freq_map.end(); it++) {
cout << it->first << " " << it->second << endl;
}
return 0;
}
```
其中,`map` 类型用于存储词频信息,`transform` 函数用于将文本转换为小写字母形式,`isalpha` 函数用于判断字符是否为字母。
相关推荐
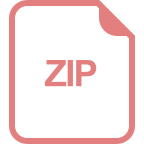
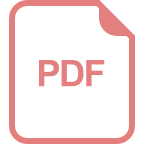














