mvc 实现切换中英文
时间: 2023-05-14 08:02:54 浏览: 98
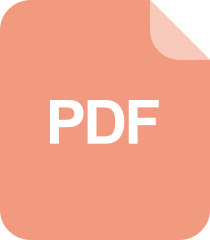
9-Spring-mvc-国际化1
MVC(Model-View-Controller)是一种常见的软件设计模式,也是实现切换中英文的一种常见的方法。具体实现步骤如下:
1. Model:定义多语言词汇表,并存储相应文本的键值对。
2. View:定义用户界面,包括各个控件的位置、大小和显示内容。
3. Controller:定义控制器,实现控件事件处理、源代码和语言文本的绑定操作。当用户切换语言时,控制器根据用户选择的语言,从词汇表中获取相应的文本,并将其绑定到对应的控件上。
具体来说,假设我们实现了一个简单的多语言登陆界面,其中包含用户名输入框和密码输入框,以及登陆按钮。首先,在 Model 中定义了如下词汇表:
```
Key Value
"username_label" "用户名:"
"password_label" "密码:"
"login_button" "登陆"
"switch_language_button" "切换语言"
```
接下来,在 View 中定义了如下控件并绑定相应的位置、大小和初始文本:
```
Label usernameLabel = new Label("用户名:");
TextField usernameField = new TextField();
Label passwordLabel = new Label("密码:");
PasswordField passwordField = new PasswordField();
Button loginButton = new Button("登陆");
Button switchLanguageButton = new Button("切换语言");
```
最后,在 Controller 中实现了控件事件处理和语言文本的绑定操作,示例代码如下:
```
public class LoginController {
private LoginModel model;
private LoginView view;
private Locale currentLocale;
public LoginController(LoginModel model, LoginView view) {
this.model = model;
this.view = view;
this.currentLocale = Locale.getDefault();
bindText();
bindEvents();
}
private void bindText() {
ResourceBundle bundle = ResourceBundle.getBundle("messages", currentLocale);
view.getUsernameLabel().setText(bundle.getString("username_label"));
view.getPasswordLabel().setText(bundle.getString("password_label"));
view.getLoginButton().setText(bundle.getString("login_button"));
view.getSwitchLanguageButton().setText(bundle.getString("switch_language_button"));
}
private void bindEvents() {
view.getSwitchLanguageButton().setOnAction(event -> {
if (currentLocale.equals(Locale.ENGLISH)) {
currentLocale = Locale.CHINESE;
} else {
currentLocale = Locale.ENGLISH;
}
bindText();
});
view.getLoginButton().setOnAction(event -> {
// 登陆按钮事件处理
...
});
}
}
```
在 Controller 中,我们通过 ResourceBundle 类从词汇表中获取当前语言下的文本,并将其绑定到 View 对应的控件上。同时,我们还实现了一个切换语言按钮,当用户点击该按钮时,Controller 根据当前语言选择下一个语言,并重新绑定语言文本到控件上。
通过上述方式,我们就实现了一个简单的多语言登陆界面,用户可以通过切换语言按钮,自由选择中英文进行登陆操作。
阅读全文
相关推荐
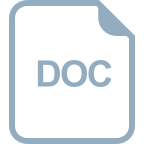
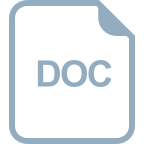
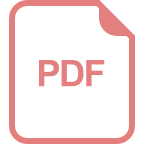
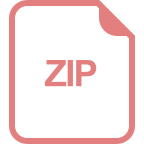
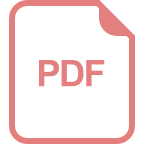
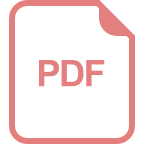
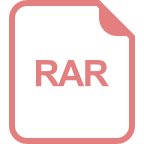
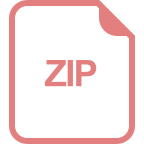
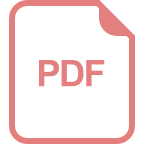
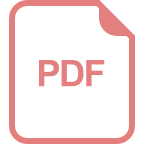
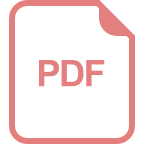
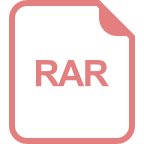
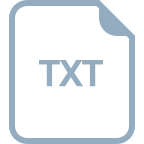
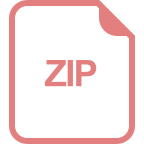
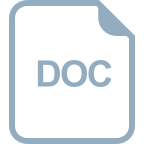
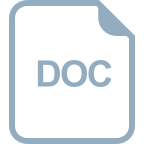
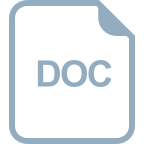