stm32f103c8t6用pid算法控制五路循迹小车
时间: 2023-08-24 09:08:05 浏览: 62
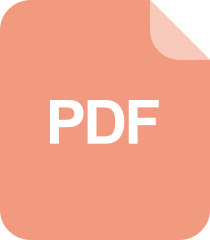
PID控制算法来控制职能小车

循迹小车的五路传感器可以检测到地面黑白交替的情况,根据传感器的数据可以得到小车的位置信息。PID算法可以根据位置信息计算出小车需要调整的速度和方向,从而实现自动控制。下面是一个简单的代码框架,可以供您参考:
```c
#include "stm32f10x.h"
#define Sensor1_GPIO GPIOA
#define Sensor1_Pin GPIO_Pin_0
#define Sensor2_GPIO GPIOA
#define Sensor2_Pin GPIO_Pin_1
#define Sensor3_GPIO GPIOA
#define Sensor3_Pin GPIO_Pin_2
#define Sensor4_GPIO GPIOA
#define Sensor4_Pin GPIO_Pin_3
#define Sensor5_GPIO GPIOA
#define Sensor5_Pin GPIO_Pin_4
#define Motor1_GPIO GPIOB
#define Motor1_EN_Pin GPIO_Pin_0
#define Motor1_IN1_Pin GPIO_Pin_1
#define Motor1_IN2_Pin GPIO_Pin_2
#define Motor2_GPIO GPIOB
#define Motor2_EN_Pin GPIO_Pin_10
#define Motor2_IN1_Pin GPIO_Pin_11
#define Motor2_IN2_Pin GPIO_Pin_12
float Kp = 1.0;
float Ki = 0.0;
float Kd = 0.0;
float last_error = 0.0;
float integral = 0.0;
void Motor1_Forward(void)
{
GPIO_SetBits(Motor1_GPIO, Motor1_IN1_Pin);
GPIO_ResetBits(Motor1_GPIO, Motor1_IN2_Pin);
}
void Motor1_Backward(void)
{
GPIO_ResetBits(Motor1_GPIO, Motor1_IN1_Pin);
GPIO_SetBits(Motor1_GPIO, Motor1_IN2_Pin);
}
void Motor2_Forward(void)
{
GPIO_SetBits(Motor2_GPIO, Motor2_IN1_Pin);
GPIO_ResetBits(Motor2_GPIO, Motor2_IN2_Pin);
}
void Motor2_Backward(void)
{
GPIO_ResetBits(Motor2_GPIO, Motor2_IN1_Pin);
GPIO_SetBits(Motor2_GPIO, Motor2_IN2_Pin);
}
void Motor1_Stop(void)
{
GPIO_ResetBits(Motor1_GPIO, Motor1_IN1_Pin | Motor1_IN2_Pin);
}
void Motor2_Stop(void)
{
GPIO_ResetBits(Motor2_GPIO, Motor2_IN1_Pin | Motor2_IN2_Pin);
}
int main(void)
{
// 初始化传感器和电机
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_GPIOB, ENABLE);
GPIO_InitStructure.GPIO_Pin = Sensor1_Pin | Sensor2_Pin | Sensor3_Pin | Sensor4_Pin | Sensor5_Pin;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_Init(Sensor1_GPIO, &GPIO_InitStructure);
GPIO_Init(Sensor2_GPIO, &GPIO_InitStructure);
GPIO_Init(Sensor3_GPIO, &GPIO_InitStructure);
GPIO_Init(Sensor4_GPIO, &GPIO_InitStructure);
GPIO_Init(Sensor5_GPIO, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = Motor1_EN_Pin | Motor1_IN1_Pin | Motor1_IN2_Pin;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(Motor1_GPIO, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = Motor2_EN_Pin | Motor2_IN1_Pin | Motor2_IN2_Pin;
GPIO_Init(Motor2_GPIO, &GPIO_InitStructure);
// 初始化PID参数
float error = 0.0;
float derivative = 0.0;
float output = 0.0;
// 进入主循环
while (1)
{
// 读取传感器数据
uint8_t s1 = GPIO_ReadInputDataBit(Sensor1_GPIO, Sensor1_Pin);
uint8_t s2 = GPIO_ReadInputDataBit(Sensor2_GPIO, Sensor2_Pin);
uint8_t s3 = GPIO_ReadInputDataBit(Sensor3_GPIO, Sensor3_Pin);
uint8_t s4 = GPIO_ReadInputDataBit(Sensor4_GPIO, Sensor4_Pin);
uint8_t s5 = GPIO_ReadInputDataBit(Sensor5_GPIO, Sensor5_Pin);
// 计算位置偏差
error = (s1 * -2.0 + s2 * -1.0 + s3 * 0.0 + s4 * 1.0 + s5 * 2.0) / (s1 + s2 + s3 + s4 + s5);
// 计算积分项
integral += error;
// 计算微分项
derivative = error - last_error;
last_error = error;
// 计算输出值
output = Kp * error + Ki * integral + Kd * derivative;
// 根据输出值控制电机
if (output > 0.0)
{
Motor1_Forward();
Motor1_GPIO->BRR = Motor1_EN_Pin;
Motor2_Forward();
Motor2_GPIO->BRR = Motor2_EN_Pin;
}
else if (output < 0.0)
{
Motor1_Backward();
Motor1_GPIO->BRR = Motor1_EN_Pin;
Motor2_Backward();
Motor2_GPIO->BRR = Motor2_EN_Pin;
}
else
{
Motor1_Stop();
Motor1_GPIO->BSRR = Motor1_EN_Pin;
Motor2_Stop();
Motor2_GPIO->BSRR = Motor2_EN_Pin;
}
}
}
```
需要注意的几个点:
1. 该代码使用了GPIO模拟PWM方式控制电机,需要在初始化时设置输出频率和占空比。
2. 微调PID参数可以获得更好的控制效果。
3. 该代码仅供参考,实际应用中可能需要根据具体情况进行修改。
阅读全文
相关推荐


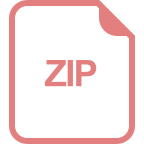
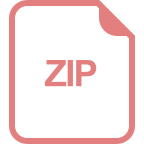
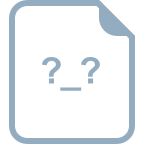
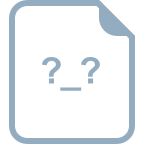
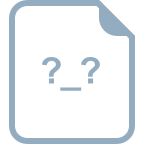