vue3高德地图添加标点
时间: 2023-11-07 11:58:39 浏览: 122
以下是使用Vue3和高德地图API添加标点的示例代码:
1. 安装高德地图API
在Vue项目中使用高德地图API需要先安装官方提供的插件:
```
npm install vue-amap --save
```
2. 注册插件
在main.js中注册插件:
```
import VueAMap from 'vue-amap';
Vue.use(VueAMap);
VueAMap.initAMapApiLoader({
key: 'YOUR_AMAP_KEY',
plugin: [
'AMap.Geocoder',
'AMap.Marker'
],
v: '1.4.15',
uiVersion: '1.0.11'
});
```
其中,YOUR_AMAP_KEY需要替换成你自己的高德地图API的key。
3. 添加地图组件
在页面中添加地图组件:
```
<template>
<div>
<div id="map"></div>
</div>
</template>
<script>
export default {
name: "Map",
mounted() {
this.initMap();
},
methods: {
initMap() {
this.$amap.initAMapApi().then(() => {
const map = new window.AMap.Map("map", {
zoom: 10,
center: [116.397428, 39.90923]
});
const marker = new window.AMap.Marker({
position: [116.397428, 39.90923]
});
marker.setMap(map);
});
}
}
};
</script>
```
其中,$amap是VueAMap插件提供的对象,initAMapApi()方法会返回一个Promise,当高德地图API加载完成后会resolve。
4. 添加标点
在地图初始化完成后,可以使用AMap.Marker添加标点:
```
const marker = new window.AMap.Marker({
position: [116.397428, 39.90923]
});
marker.setMap(map);
```
其中,position属性指定标点的经纬度坐标,setMap方法将标点添加到地图上。
完整示例代码:
```
<template>
<div>
<div id="map"></div>
</div>
</template>
<script>
export default {
name: "Map",
mounted() {
this.initMap();
},
methods: {
initMap() {
this.$amap.initAMapApi().then(() => {
const map = new window.AMap.Map("map", {
zoom: 10,
center: [116.397428, 39.90923]
});
const marker = new window.AMap.Marker({
position: [116.397428, 39.90923]
});
marker.setMap(map);
});
}
}
};
</script>
<style>
#map {
height: 500px;
}
</style>
```
阅读全文
相关推荐
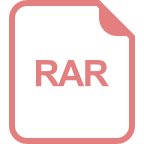






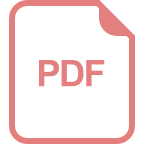
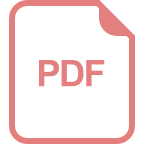

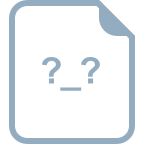
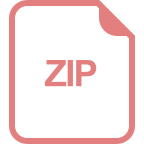

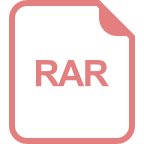
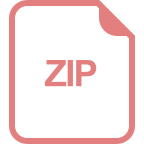
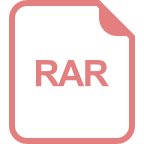