用c语言构建一个有序链表
时间: 2023-12-03 19:47:42 浏览: 28
首先,需要定义一个链表节点的结构体,包含数据和指向下一个节点的指针。
```c
struct ListNode {
int data;
struct ListNode *next;
};
```
接下来,我们定义一个函数 `insertNode`,用于将一个新节点按照顺序插入到链表中。假设链表已经按照从小到大的顺序排好了,那么我们只需要从链表的头节点开始遍历,找到第一个比新节点大的节点,然后将新节点插入到它的前面即可。
```c
void insertNode(struct ListNode **head, int data) {
struct ListNode *newNode = (struct ListNode*)malloc(sizeof(struct ListNode));
newNode->data = data;
newNode->next = NULL;
if (*head == NULL || data < (*head)->data) {
newNode->next = *head;
*head = newNode;
return;
}
struct ListNode *current = *head;
while (current->next != NULL && current->next->data < data) {
current = current->next;
}
newNode->next = current->next;
current->next = newNode;
}
```
最后,我们可以写一个简单的 `main` 函数来测试 `insertNode` 函数的正确性。
```c
int main() {
struct ListNode *head = NULL;
insertNode(&head, 2);
insertNode(&head, 4);
insertNode(&head, 1);
insertNode(&head, 3);
struct ListNode *current = head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
// 输出结果:1 2 3 4
return 0;
}
```
相关推荐
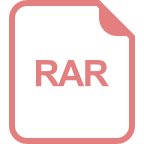
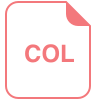
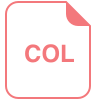
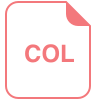











